What is Zenefits?
TriNet Zeneits is a cloud-based human resources (HR) platform that streamlines HR functions for small and medium-sized businesses (SMBs). Following its acquisition by TriNet, Zenefits has expanded its offerings to provide a comprehensive suite of HR services, including benefits administration, payroll processing, compliance management, and employee engagement tools.
In this guide, we’ll explore how to integrate Zenefits API into your applications, look at common use cases, and offer best practices for troubleshooting API issues. Whether you’re a developer, an HR-tech professional, or part of a tech team evaluating Zenefits, this blog aims to help you leverage the API effectively.
Note: This is an educational article on Zenefits API. If you’re looking for an easier way to launch integrations with Zenefits, check out Bindbee.
Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
That said, here’s what we’ll cover in this article:
- A step-by-step Zenefits API integration guide using Direct API and other key methodologies.
- An overview of the API’s capabilities and key features.
- Best practices and troubleshooting tips for easier API operations.
What is Zenefits API?
The Zenefits API is a RESTful API that allows developers to integrate and manage employee data, payroll & benefits data from the Zenefits platform.
Key Features of the Zenefits API
Architecture
- Zenefits API adheres to REST principles, enabling interaction with resources via standard HTTP methods (GET, POST, PUT, DELETE).
Authentication
- The API employs OAuth2 for secure authentication, allowing third-party applications to access user data without exposing passwords.
- Developers receive a unique Client ID and Client Secret to authenticate their applications when interacting with the Zenefits API.
Key Endpoints
- Pagination and Filtering: Supports up to 100 records per response. Use
starting_after
orending_before
query parameters to optimize data retrieval. - Webhooks: Real-time notifications for data changes like employee creation or updates help keep external systems in sync.
Use Cases
- Employee Management: Developers can create applications to manage employee data, including onboarding new hires or updating employee records.
- Benefits Administration: Integrate functionalities for managing health insurance and retirement plans within business applications.
- Payroll Processing: Automate payroll tasks by integrating payroll data with financial systems through the API.
- Compliance Tracking: Use the API to ensure labor law compliance by tracking and maintaining necessary employee information.
Zenefits API Integration Guide
We’ll walk you through two key methodologies to integrate with Namely API.
Method 1 - Direct API Integration
Step 1: Set Up Your Developer Account
- Create a Developer Account: Contact Zenefits to set up a developer account. This provides access to the sandbox environment, which is essential for testing your integration.
- Obtain Credentials: Once your account is ready, you'll receive a Client ID and Client Secret required for OAuth2 authentication.
Step 2: Understand API Authentication
- OAuth2 Authentication: Zenefits uses OAuth2 for secure API access:
- Redirect users to the Zenefits authorization URL to request permissions.
- After permission is granted, the user is redirected back to your application with an authorization code.
- Exchange the authorization code for an access token using your Client ID and Client Secret.
Step 3: Explore API Endpoints
Familiarize yourself with the following key API endpoints:
Step 4: Make API Requests
Test your API requests using tools like Postman or cURL:
curl -X GET "<https://api.zenefits.com/core/companies>" \\
-H "Authorization: Bearer YOUR_ACCESS_TOKEN"
This helps you ensure your API calls are correctly formatted and return the expected responses.
Step 5: Implement Error Handling
- 401 Unauthorized: Check if your access token has expired or if your credentials are correct.
- 404 Not Found: Ensure the requested resource (like an employee ID) exists.
- 500 Internal Server Error: Retry the request or escalate to Zenefits support if the issue persists.
Step 6: Set Up Webhooks (Optional)
To receive real-time updates on changes within Zenefits (e.g., employee creation or updates):
- Register a Webhook Endpoint: Set up an endpoint in your application to receive event notifications.
- Subscribe to Events: Use the Zenefits API to subscribe to relevant events for continuous data synchronization.
Method 2 - SDKs and Libraries Integration
Using SDKs provided by Zenefits or third-party platforms can simplify the integration process, especially for developers looking to reduce development time and complexity.
Step 1: Choose an SDK
Select the appropriate SDK based on your development environment:
- Node.js
- Python
- PHP
- .NET
Step 2: Install the SDK
For example, to install the Node.js SDK:
npm install @bindbee/bindbee-hris-node
Step 3: Initialize the SDK
Once installed, initialize the SDK within your application:
const BindbeeHRIS = require('@bindbeeapi/bindbee-hris-node');
const client = new BindbeeHRIS.Client({
apiKey: 'YOUR_API_KEY',
});
Step 4: Make API Calls Using the SDK
The SDK provides pre-built methods for easy API interaction. For example:
client.companiesList()
.then(companies => {
console.log(companies);
})
.catch(error => {
console.error('Error fetching companies:', error);
});
client.employeesList()
.then(employees => {
console.log(employees);
})
.catch(error => {
console.error('Error fetching employees:', error);
});
Step 5: Testing and Deployment
- Test Thoroughly: Perform rigorous testing in the sandbox environment to ensure your integration works as expected.
- Deploy to Production: Once testing is complete, deploy your integration to the production environment.
Method 3: Integrating with Zenefits Using Bindbee
Overview - Bindbee is a unified API platform that lets you integrate with Zenefits (and 50+ HRIS, ATS & Payroll Platforms) in just a few minutes. No-code, zero-maintenance integrations that offers round-the-clock data sync at one-tenth the cost of custom Integrations. Check out Bindbee today!
Setting up Zenefits Integration with Bindbee
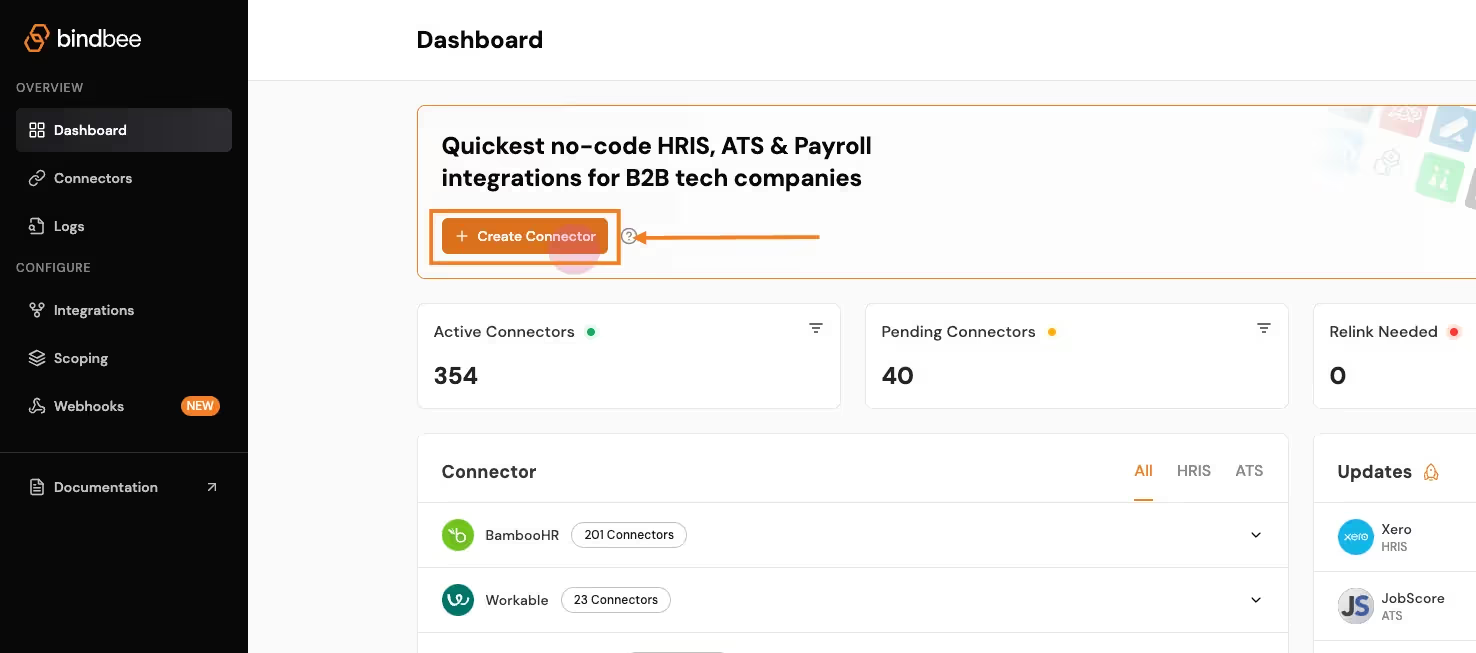
- Create a Connector:
- Click on Create Connector from the dashboard.
- Select HRIS as the type of integration. Enter customer details and give your connector a unique ID (e.g., Zenefits_Integration).
- Generate a Magic Link:
- After setting up the connector, click Create Link to generate a magic link. This will allow the customer to authenticate the connection with Zenefits API.
- Open the link and enter the necessary credentials (e.g., Zenefits key, subdomain). This step establishes the connection between the platform and Bindbee.
- Sync the Connector:
- Once the connection is made, the connector will begin syncing data from Zenefits. This may take a few minutes depending on the size of the data. You can track the sync status in the connector section.
- Access the Synced Data:
- After syncing, go to the Employee section in the Bindbee dashboard and select Get Employees to retrieve employee data from Namely.
- Get the API Key and Connector Token:
- Copy the API key and the x-connector-token from the Bindbee dashboard, which you will need to make authenticated API requests.
Retrieving Employee Data with Bindbee
Once the Zenefits data has been synced, you can retrieve employee data on to your application via the Bindbee API.
Here’s a step-by-step process for accessing synced data from Zenefits through Bindbee’s unified API:
- Request Setup:
- Use the Bindbee API to send a request for employee data. You’ll need both your Bindbee API token and the x-connector-token.
- Example Request:
- Using Python:
import requests
url = "https://api.bindbee.com/hris/v1/employees"
headers = {
"Authorization": "Bearer YOUR_BINDBEE_API_KEY",
"x-connector-token": "YOUR_CONNECTOR_TOKEN",
"Accept": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
- Using cURL:
curl --request GET \
--url https://api.bindbee.com/hris/v1/employees \
--header 'Authorization: Bearer YOUR_BINDBEE_API_KEY' \
--header 'x-connector-token: YOUR_CONNECTOR_TOKEN'
This request will return a list of employee objects, including details like the employee’s first name, last name, job title, department, and contact information.
Sample Response:
{
"items": [
{
"id": "018b18ef-c487-703c-afd9-0ca478ccd9d6",
"first_name": "John",
"last_name": "Doe",
"job_title": "Chief Technology Officer",
"department": "Engineering",
"work_email": "john@johndoe.dev"
}
]
}
Bulk Employee Data Retrieval
For retrieving large datasets, Bindbee simplifies the process by allowing you to fetch bulk employee data from Sage.
The pagination feature helps manage large responses by returning results in pages.
What is Pagination?
“Pagination is the process of dividing a large dataset into smaller, manageable chunks or pages, allowing users to navigate through the data more easily without overwhelming them with too much information at once.”
- Pagination Parameters:
- Use the
cursor
andpage_size
parameters to navigate through the results. By default, Bindbee returns 50 records per page. - Example of a paginated request:
- Use the
url = "https://api.bindbee.com/hris/v1/employees?cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1&page_size=50"
response = requests.get(url, headers=headers)
- Querying for Specific Employee Data:
- You can further refine your request by filtering based on specific fields, such as manager_id, remote_id, or company_id to get employees under a particular manager or company.
Check out the entire documentation here - Bindbee’s API documentation.
Get Started with Zenefits API Using Bindbee
Integrating with Zenefits shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink—draining valuable engineering resources.
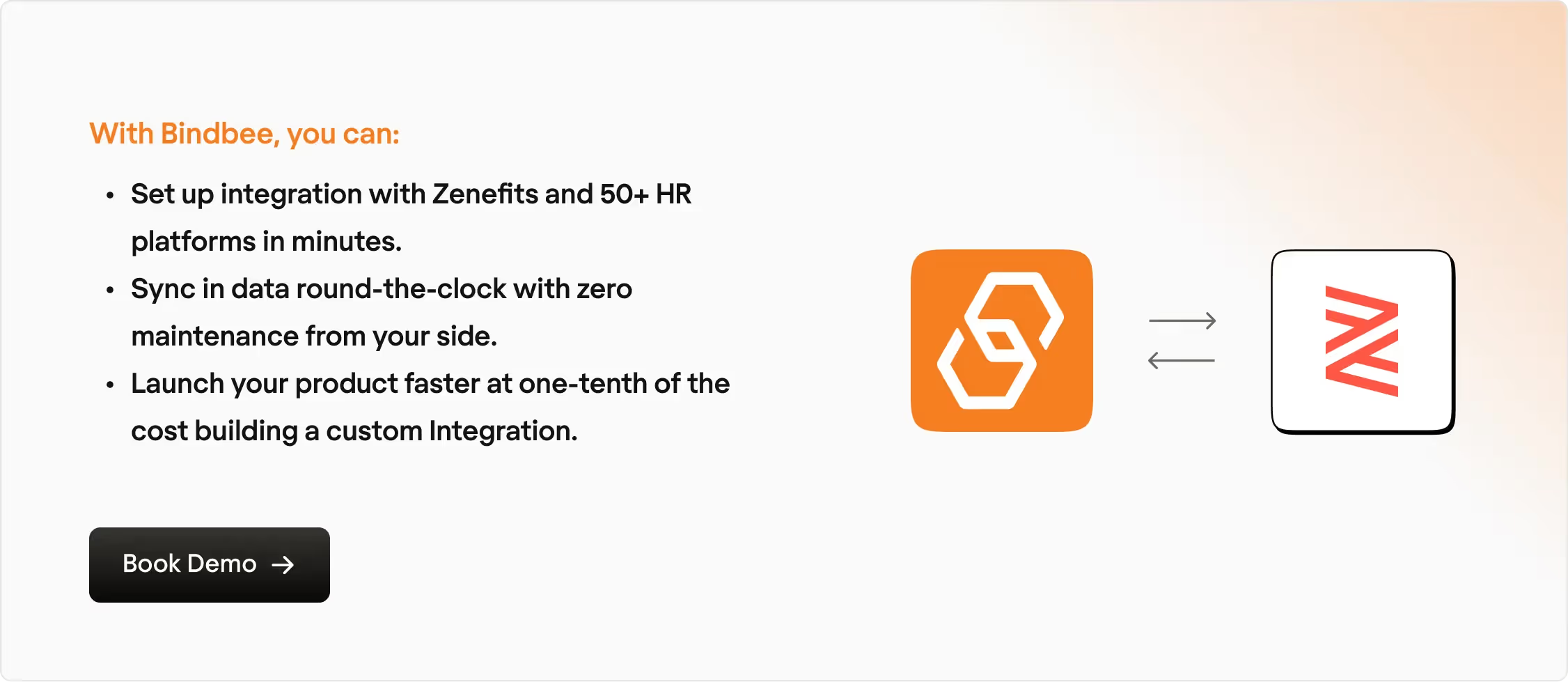
Let us handle the heavy lifting. You focus on growth, what say?
Book a demo with our experts today.