What is UKG Pro?
UKG is an human capital management (HCM) solution designed to streamline payroll, human resources (HR), talent management, and employee engagement.
In this guide, we’ll explore how to integrate UKG API into your applications, look at common use cases, and offer best practices for troubleshooting API issues. Whether you’re a developer, HR-tech professional, or part of a tech team evaluating UKG, this blog aims to help you leverage the API effectively.
Note - This is an educational article on UKG API. If you’re looking for an easier way to launch integrations with UKG, check out Bindbee.
Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
That said, Here’s what we’ll cover in this article:
- A step-by-step UKG integration guide using Direct API, SDKs and Bindbee.
- An overview of the API’s capabilities and key features.
- Best practices and troubleshooting tips for smooth API operations.
Understanding UKG API
What is UKG API?
The UKG API is a RESTful service that enables developers to programmatically access and manipulate data within the UKG platform.
This includes managing employee records, time and attendance, scheduling, and payroll information. The UKG API facilitates integration with other applications, enhancing workforce management and operational efficiency.
API Authentication
The UKG API utilizes two primary authentication methods to ensure secure access:
- OAuth 2.0: This is the preferred method for authenticating API requests. It allows for secure, token-based access, providing granular control over data access.
- Example of authenticating using OAuth 2.0 with
curl
:
curl -X POST "<https://api.ukg.com/oauth/token>" \\
-H "Content-Type: application/x-www-form-urlencoded" \\
-d "grant_type=client_credentials&client_id={client_id}&client_secret={client_secret}"
- API Keys: In some cases, UKG may provide API keys for simpler integrations. These keys should be included in the headers of your requests.
Handling API Requests
API requests to the UKG system follow the REST structure, utilizing standard HTTP methods (GET, POST, PUT, DELETE) to interact with resources. Responses are typically returned in JSON format.
- Base URL:
<https://api.ukg.com/v1/>
UKG API - Key Use Cases
- Employee Management: Access and modify employee records, including personal details and job roles.
- Time and Attendance: Manage time-off requests, clock-ins/outs, and attendance records.
- Scheduling: Create and manage employee schedules efficiently.
- Payroll Processing: Integrate payroll data for accurate compensation calculations.
- Reporting: Generate custom reports based on various workforce metrics.
UKG API Integration Guide
We’ll walk through three methodologies: Direct API, SDKs, and Bindbee.
Whether you’re comfortable with code, prefer a more simplified route for integration (SDKs), or wants to outsource the entire process to a unified API like Bindbee, this section has you covered.
1. Direct API Integration
Overview: Direct API integration involves manually using the UKG Pro API endpoints in your application code.
This method gives developers full control over how the API is used, offering the most flexibility but also requiring a deeper understanding of the API's architecture and handling all aspects of the integration process.
Direct API Integration - Step-by-Step Breakdown
Authentication:
Secure access to the API must be established using OAuth 2.0, which is the primary authentication method for accessing the UKG Pro API.
- OAuth 2.0: OAuth 2.0 provides more granular control over access by generating access tokens based on the permissions assigned to the requesting user or application. This is the recommended method for accessing the UKG Pro API.
Example for OAuth 2.0 token generation:
curl -X POST <https://HOSTNAME/ukgpro/oauth/token> \\
-H 'appkey: YOUR_APP_KEY' \\
-H 'Content-Type: application/x-www-form-urlencoded' \\
-d 'grant_type=password&username=USERNAME&password=PASSWORD&client_id=CLIENT_ID&client_secret=CLIENT_SECRET'
Important Note: The endpoint and parameters may vary based on your specific UKG Pro implementation. Always refer to the official UKG Pro API documentation for the correct endpoint and token generation process.
After obtaining the access token, include it in the Authorization header for each API call, using the format Bearer {access_token}
.
Making API Calls:
Interact with the UKG Pro API by making HTTP requests such as GET, POST, PUT, DELETE to access or update employee data, payroll details, and attendance management records.
Example 1: Retrieve Employee Data
Here’s how you can pull data for a specific employee using the UKG Pro API.
import requests
url = "<https://api.ukgpro.com/v1/employees/4?fields=firstName,lastName>"
headers = {
"Accept": "application/json",
"Authorization": "Bearer {access_token}"
}
response = requests.get(url, headers=headers)
print(response.json())
This code retrieves the employee’s first name and last name.
You can easily expand the fields to include additional employee information, such as their job title, department, or location.
Expanded Example:
url = "<https://api.ukgpro.com/v1/employees/4?fields=firstName,lastName,jobTitle,department>"
response = requests.get(url, headers=headers)
print(response.json())
This will return not only the employee’s name but also their job title and the department they belong to, making it useful for more detailed employee data retrieval.
Retrieve Bulk Employee Data (Custom Reports):
If you need to retrieve data for multiple employees or generate a custom report, you can use the bulk data retrieval endpoint offered by the UKG Pro API.
Example of generating a custom employee report:
url = "<https://api.ukgpro.com/v1/custom/reports?format=JSON>"
payload = {
"fields": ["firstName", "lastName", "jobTitle", "department"]
}
response = requests.post(url, json=payload, headers=headers)
print(response.json())
This API call will return the first name, last name, job title, and department of multiple employees in JSON format. The specifics of this endpoint (like available fields and report formats) can vary, so it's essential to verify the exact endpoint structure in the official UKG Pro API documentation.
Response Handling
The UKG Pro API returns JSON responses, which must be parsed and processed within your application.
Ensure proper error handling for different HTTP status codes (e.g., 200 OK, 400 Bad Request, 500 Internal Server Error) and parse JSON data to extract meaningful information for further use.
Example for handling a successful response:
if response.status_code == 200:
employee_data = response.json()
print("Employee Data:", employee_data)
else:
print(f"Error: {response.status_code}")
The above code checks if the response was successful (HTTP 200), and if so, it processes the employee data.
Otherwise, it logs the error code. Always ensure that error messages are handled appropriately and reported accurately.
Benefits of Direct API Integration
- Full control over API usage: Direct integration allows you to customize every aspect of how the API is accessed and interacted with.
- Custom error handling: Directly manage how errors are caught and resolved within your application.
- Flexibility: Developers can build custom workflows that suit their specific needs, utilizing all available API endpoints.
2. Using SDKs
Overview: For developers looking to simplify the process, using Software Development Kits (SDKs) provided by UKG or third-party platforms might be a well-off alternative.
SDKs abstract away many complexities involved in manual API calls, offering pre-built functions to handle common tasks like authentication and API requests.
Benefits:
- Reduced Development Time: SDKs come with pre-built functions that handle common tasks, such as authentication and API calls, allowing developers to focus on building the application logic.
- User-friendly interface: The SDK abstracts many of the complexities of working with the API directly, providing an easier interface for developers.
- Automatic updates: SDKs are often kept up to date with the latest API changes, meaning your integration remains compatible without extensive code modifications.
UKG - SDK Integration Guide
1. Install the SDK
Begin by installing the SDK for your preferred programming language.
SDKs often come with built-in methods for handling API requests and responses, simplifying the development process.
Example (for a Python SDK):
pip install ukgpro-sdk
2.Authentication Handling
SDKs often manage the authentication process automatically.
Once the SDK is configured with your API keys or OAuth tokens, you can start making API calls without worrying about manually setting up authorization headers for each request.
Example:
from ukgpro_sdk import UKGPro
# Authenticate
client = UKGPro(api_key="YOUR_API_KEY")
# Fetch employee data
employees = client.get_employees()
3. Making API Calls:
Instead of constructing raw HTTP requests, SDKs provide high-level functions that handle the underlying HTTP calls.
For example, to retrieve employee data, the SDK might offer a method like get_employees()
that abstracts away the complexities of building the HTTP request manually.
4. Error Handling & Response Parsing
SDKs typically offer built-in error handling, making it easier to catch and respond to errors without manually checking HTTP status codes or parsing raw JSON data.
Method 3: Bindbee
Overview - Bindbee is a unified API platform that lets you integrate with UKG (and 50+ HRIS, ATS & Payroll Platforms) in just a few minutes. No-code, zero-maintenance integrations that offers round-the-clock data sync at one-tenth the cost of custom Integrations. Check out Bindbee today!
Setting up UKG Integration with Bindbee
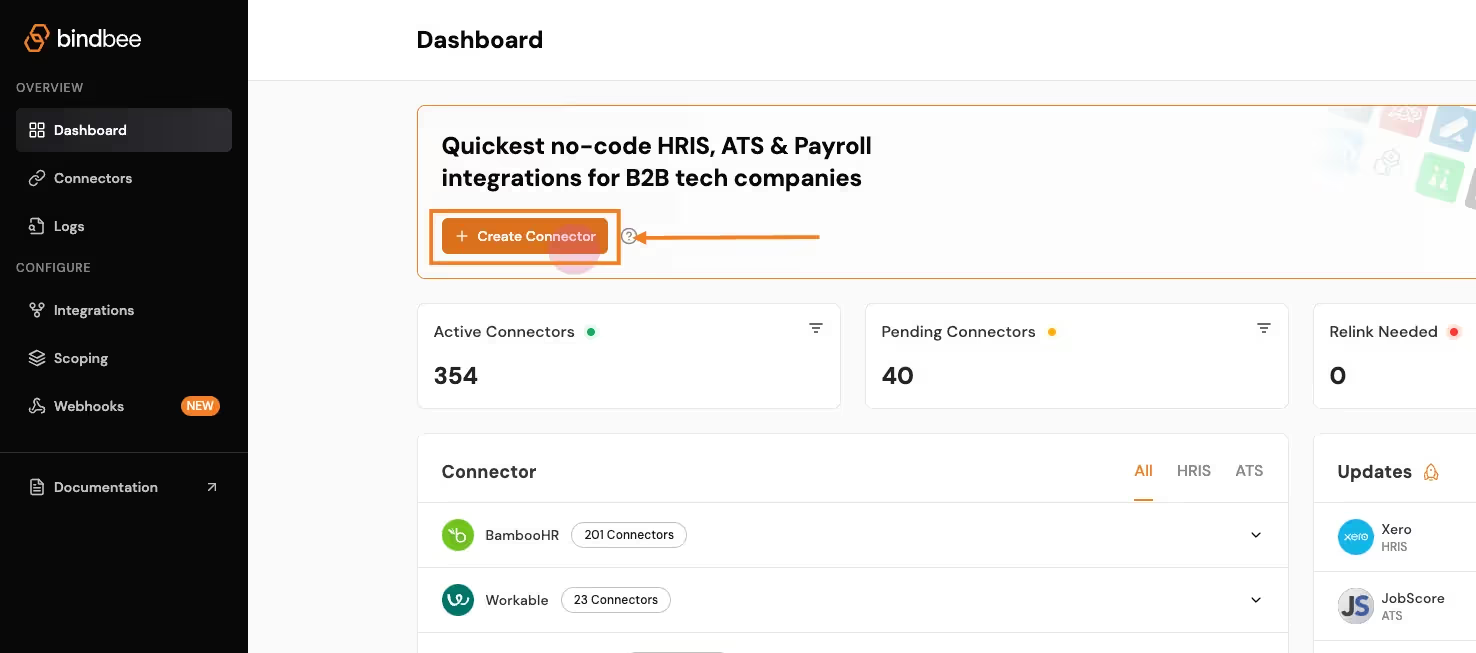
- Create a Connector:
- Click on Create Connector from the dashboard.
- Select HRIS as the type of integration. Enter customer details and give your connector a unique ID (e.g., UKGPro_Integration).
- Generate a Magic Link:
- After setting up the connector, click Create Link to generate a magic link. This will allow the customer to authenticate the connection with UKG.
- Open the link and enter the necessary credentials (e.g., UKG API key, subdomain). This step establishes the connection between UKG and Bindbee.
- Sync the Connector:
- Once the connection is made, the connector will begin syncing data from UKG. This may take a few minutes depending on the size of the data. You can track the sync status in the connector section.
- Access the Synced Data:
- After syncing, go to the Employee section in the Bindbee dashboard and select Get Employees to retrieve employee data from UKG.
- Get the API Key and Connector Token:
- Copy the API key and the x-connector-token from the Bindbee dashboard, which you will need to make authenticated API requests.
Retrieving Employee Data with Bindbee
Once the UKG data has been synced, you can retrieve employee data on to your application via the Bindbee API.
Here’s a step-by-step process for accessing synced data from UKG through Bindbee’s unified API:
- Request Setup:
- Use the Bindbee API to send a request for employee data. You’ll need both your Bindbee API token and the x-connector-token.
- Example Request:
- Using Python:
import requests
url = "https://api.bindbee.com/hris/v1/employees"
headers = {
"Authorization": "Bearer YOUR_BINDBEE_API_KEY",
"x-connector-token": "YOUR_CONNECTOR_TOKEN",
"Accept": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
- Using cURL:
curl --request GET \
--url https://api.bindbee.com/hris/v1/employees \
--header 'Authorization: Bearer YOUR_BINDBEE_API_KEY' \
--header 'x-connector-token: YOUR_CONNECTOR_TOKEN'
This request will return a list of employee objects, including details like the employee’s first name, last name, job title, department, and contact information.
Sample Response:
{
"items": [
{
"id": "018b18ef-c487-703c-afd9-0ca478ccd9d6",
"first_name": "John",
"last_name": "Doe",
"job_title": "Chief Technology Officer",
"department": "Engineering",
"work_email": "john@johndoe.dev"
}
]
}
Bulk Employee Data Retrieval
For retrieving large datasets, Bindbee simplifies the process by allowing you to fetch bulk employee data from UKG.
The pagination feature helps manage large responses by returning results in pages.
What is Pagination?
“Pagination is the process of dividing a large dataset into smaller, manageable chunks or pages, allowing users to navigate through the data more easily without overwhelming them with too much information at once.”
- Pagination Parameters:
- Use the
cursor
andpage_size
parameters to navigate through the results. By default, Bindbee returns 50 records per page. - Example of a paginated request:
- Use the
url = "https://api.bindbee.com/hris/v1/employees?cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1&page_size=50"
response = requests.get(url, headers=headers)
- Querying for Specific Employee Data:
- You can further refine your request by filtering based on specific fields, such as manager_id, remote_id, or company_id to get employees under a particular manager or company.
Debugging and Best Practices
When working with APIs for UKG, you might encounter various errors during integration.
These issues typically arise from authentication, permissions, or rate limits.
Here's a breakdown of common errors, their causes, and solutions.
Here’s a comprehensive and detailed table for Debugging and Best Practices when working with the UKG API:
UKG API - Best Practices for Efficient API Usage
To avoid common pitfalls and ensure your UKG integration performs efficiently, follow these guidelines:
Efficient Data Syncing
- Batch Requests: When retrieving large datasets, use bulk endpoints like custom reports to minimize the number of API calls.
- Pagination: For large data sets, paginate responses to handle records in manageable batches, ensuring your integration remains performant and within rate limits.
Example:
url = "<https://api.ukg.com/v1/employees?page_size=50&cursor=YOUR_CURSOR>"
response = requests.get(url, headers=headers)
Handle Rate Limits Gracefully
- Use HTTP headers to monitor rate limits. UKG returns
Retry-After
headers when limits are exceeded, indicating when to retry. - Implement exponential backoff to delay retries progressively in case of transient errors (e.g., 429 Too Many Requests or 503 Service Unavailable).
Example of exponential backoff:
import time
for i in range(5): # Retry up to 5 times
response = requests.get(url, headers=headers)
if response.status_code == 200:
break
elif response.status_code == 429:
retry_after = int(response.headers.get('Retry-After', 10))
time.sleep(retry_after * (2 ** i)) # Exponentially increase retry time
Error Handling
- Always check the status codes and handle errors appropriately. Use logging to capture errors for debugging.
- For critical errors like 401 Unauthorized or 403 Forbidden, alert the user immediately to correct the API key or permissions.
Caching
- For frequently accessed data, implement caching mechanisms to reduce repetitive API calls and improve performance.
- For example, cache employee data and refresh it periodically instead of fetching it on every request.
- Timeouts and Retries:
- Set a reasonable timeout for API requests to avoid hanging indefinitely. Implement retries with a timeout threshold to gracefully handle network issues or server delays.
Example:
response = requests.get(url, headers=headers, timeout=10) # Timeout after 10 seconds
Use the Right HTTP Methods
- Follow RESTful best practices by using the correct HTTP methods:
GET
for retrieving data.POST
for creating new resources.PUT
orPATCH
for updating existing resources.DELETE
for deleting resources.
Monitoring and Alerts
- Set up monitoring for your API integration to track response times, error rates, and downtime. This will help you identify potential issues before they impact users.
- Use tools like Postman or API monitoring platforms to regularly test and validate your API requests.
By applying these best practices and handling common errors effectively, your UKG integration will remain efficient, reliable and robust.
UKG API Documentation and Resources
- UKG API Documentation
- Tools for testing and debugging:
- Bindbee’s API documentation.
Get Started with UKG API Using Bindbee
Integrating with UKG shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink—draining valuable engineering resources.

Let us handle the heavy lifting. You focus on growth, what say?
Book a demo with our experts today.