window.postMessage() is part of the Window interface in web browsers. It allows for controlled, secure messaging between windows or frames that may not share the same origin (protocol, host, and port).
When developing modern web applications involving multiple windows or iframes, establishing secure communication between them is a challenge, particularly when crossing domains.
At Bindbee, this has been proven crucial for our Embed feature, where an iframe must communicate with its parent window across different domains.
In this guide, we’ll dive into how you can leverage window.postMessage() for efficient communication between windows, focusing on practical implementations and best practices.
Why Cross-Origin Communication is a Challenge
The Same-Origin Policy is a fundamental web security feature designed to prevent unauthorized access between resources from different origins (domains, protocols, or ports).
While this policy helps with improving security, it can complicate legitimate cross-origin communication, such as when you need to pass data between a parent window and an embedded iframe from a different origin.
Real-World Example
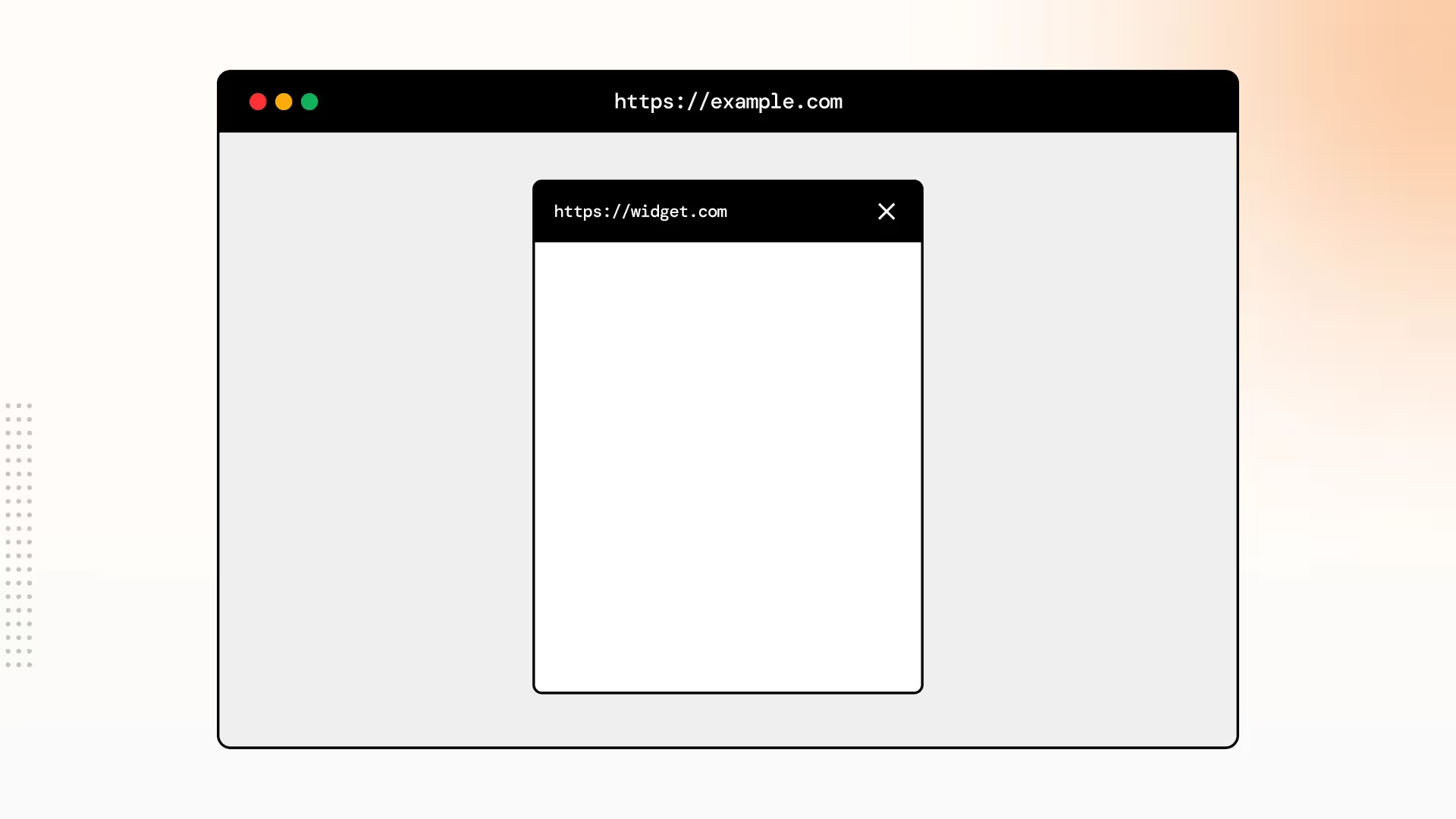
Consider the following scenario:
- You have a website,
https://example.com
, embedding content from another domain,https://widget.com
, through an iframe. - The iframe content must exchange data with the parent window, but direct communication is blocked by the Same-Origin Policy.
This is where window.postMessage() comes into play, offering a safe workaround for cross-origin communication.
What is window.postMessage()
?
window.postMessage()
is a method designed to facilitate communication between windows or iframes, regardless of their origin.
It allows one window (the sender) to send a message to another (the receiver), with built-in controls to ensure that communication remains safe.
Basic Syntax:
targetWindow.postMessage(message, targetOrigin, [transfer]);
- targetWindow: The window object to which you want to send the message.
- message: The data you want to send, which can be any serializable data type (objects, strings, etc.).
- targetOrigin: A critical security parameter that specifies the expected origin of the receiving window. Always set this explicitly for security (never use
"*"
in production). - transfer (optional): Transferable objects that can improve performance by transferring ownership rather than copying.
Receiving Messages:
The receiver window can listen for messages using an event listener for the message
event:
window.addEventListener("message", (event) => {
// Verify the sender’s origin
if (event.origin !== "https://trusted-domain.com") return;
// Handle the message
console.log("Received message:", event.data);
});
Implementing Secure Communication : Step-by-step Guide
Let’s break down how to implement window.postMessage()
for efficient communication, using a parent window and an embedded iframe as an example.
This setup ensures secure data transfer while maintaining strict control over which origins can send or receive data.
1. Setting Up the Parent Window (https://example.com
)
In the parent window, the goal is to listen for messages from the iframe and respond appropriately:
// Step 1: Listen for messages from the iframe
window.addEventListener("message", (event) => {
if (event.origin !== "https://widget.com") return; // Validate the origin
// Step 2: Process the message
if (event.data.type === "widgetHeight") {
document.getElementById("widget-iframe").style.height = `${event.data.height}px`;
}
});
// Step 3: Send a message to the iframe
function sendMessageToWidget(message) {
const iframe = document.getElementById("widget-iframe");
iframe.contentWindow.postMessage(message, "https://widget.com"); // Always specify the targetOrigin
}
2. Configuring the Embedded iframe (https://widget.com
)
On the iframe side, you can similarly set up message handling to receive data from the parent window and send messages back:
// Step 1: Listen for messages from the parent window
window.addEventListener("message", (event) => {
if (event.origin !== "https://example.com") return; // Verify the sender’s origin
// Step 2: Process the received message
if (event.data.type === "updateContent") {
updateWidgetContent(event.data.content); // Custom logic
}
});
// Step 3: Notify the parent window of iframe height
function notifyParentOfHeight() {
const height = document.body.scrollHeight;
window.parent.postMessage({ type: "widgetHeight", height: height }, "https://example.com");
}
Best Practices for Implementation
While window.postMessage()
is a powerful tool for cross-origin communication, it must be implemented with care.
Here are some best practices to ensure security:
1. Always Validate Origins:
- When receiving messages, always verify the
event.origin
to ensure that the message is coming from a trusted source. - This prevents malicious domains from sending unverified data.
if (event.origin !== "https://trusted-domain.com") return;
2. Specify targetOrigin Explicitly:
- Avoid using "*" as the targetOrigin when sending messages. Always specify the exact origin to ensure that only the intended recipient can handle the message.
iframe.contentWindow.postMessage(message, "https://widget.com");
3. Sanitize and Validate Incoming Data:
- Treat all incoming messages as untrusted.
- Validate and sanitize the data to prevent potential injection attacks or security breaches.
4. Use HTTPS for All Communication:
- Always ensure communication is happening over HTTPS to prevent man-in-the-middle (MITM) attacks.
5. Limit Privileged Actions:
- Be cautious about performing sensitive actions based on incoming messages. Where possible, implement additional authentication or authorization checks.
Troubleshooting Common Issues
While implementing cross-origin communication, you might run into common issues.
Here’s a quick guide to troubleshooting:
- Issue: Messages Not Being Received:
- Solution: Double-check the
targetOrigin
andevent.origin
values. Ensure they are correctly specified and match the intended domains.
- Solution: Double-check the
- Issue: Security Warnings or Errors:
- Solution: Verify that HTTPS is enforced on both domains and that any sensitive data is properly sanitized and validated.
- Issue: Poor Performance:
- Solution: If you are sending large objects or large amounts of data, consider using the
transfer
parameter to optimize performance by transferring ownership of transferable objects.
- Solution: If you are sending large objects or large amounts of data, consider using the
Takeaways
The window.postMessage()
method offers a flexible and powerful solution for efficient cross-origin communication in web applications.
By adhering to best practices—such as verifying origins, specifying targetOrigin
, and sanitizing data—you can implement safe and efficient message passing between windows.
Remember, secure communication is not just about making things work—it’s about making them work safely. As always, test thoroughly and prioritize security when dealing with cross-origin interactions.
Further Reading and Resources: