What is Paycor?
Paycor is a comprehensive human capital management (HCM) platform that provides a wide range of services designed to support small to midsize businesses in managing their human resources effectively.
In this guide, we’ll explore how to integrate Paycor API into your applications, look at common use cases, and offer best practices for troubleshooting API issues. Whether you’re a developer, HR-tech professional, or part of a tech team evaluating Paycor, this blog aims to help you leverage the API effectively.
Note - This is an educational article on Paycor API. If you’re looking for an easier way to launch integrations with Paycor, check out Bindbee.
Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
That said, Here’s what we’ll cover in this article:
- A step-by-step Paycor API integration guide using Direct API and other key methodologies.
- An overview of the API’s capabilities and key features.
- Best practices and troubleshooting tips for smooth API operations.
Understanding Paycor API
What is Paycor API?
Paycor API is a robust interface designed to facilitate integration between Paycor's human capital management platform and other software applications.
This API allows developers to access a wide range of HR and payroll data, enabling functionalities such as employee management, payroll processing, and benefits administration.
Paycor API - Key Features
- Data Access: The API provides endpoints for retrieving and managing various types of employee and payroll data. This includes accessing employee records, payroll statements, and benefits information.
- Authentication: To use the Paycor API, developers must authenticate via OAuth 2.0. This involves obtaining an access token using client credentials, which is then used to make authorized requests to the API.
- Integration Options: Paycor offers both a public API for direct integrations and options through third-party services like Finch, which can simplify the integration process by allowing access to multiple HR systems through a unified interface24.
- Security: The API employs industry-standard security measures, including TLS encryption for data in transit and AES encryption for data at rest, ensuring that sensitive information is protected throughout the integration process
Paycor API Integration Guide
We’ll walk you through three key methodologies to integrate with Paycor API.
Method 1: Direct API Integration
Overview: Direct API Integration allows developers to connect to Paycor's API for HR and payroll data access. This method is ideal for real-time interaction with Paycor’s data, allowing your application to make API calls and manage employee, payroll, and other HR data as needed.
Step-by-Step Guide
1. Set Up Your Development Environment
To interact with Paycor’s API, ensure that your development environment is properly configured.
You’ll need the following Python libraries for making HTTP requests and securely managing environment variables:
requests
– for making HTTP requests.python-dotenv
– for loading environment variables from a.env
file, such as API credentials.
You can install these libraries using pip:
pip install requests python-dotenv
2. Obtain API Credentials
Sign up for a Paycor developer account and generate the necessary Client ID and Client Secret from the Paycor developer portal. These credentials are required for authenticating your API calls.
Best Practice: Store these credentials in a .env file to keep them secure and separate from your codebase. This will help prevent accidental exposure of sensitive information in version control systems like Git.
3. Authenticate and Get Access Token
Use the following code snippet to authenticate your application and obtain an OAuth 2.0 access token. This token will be required in all subsequent API requests.
import requests
from dotenv import load_dotenv
import os
load_dotenv() # Load environment variables from the .env file
def get_access_token():
url = "<https://api.paycor.com/v1/authentication>"
payload = {
"client_id": os.getenv("PAYCOR_CLIENT_ID"),
"client_secret": os.getenv("PAYCOR_CLIENT_SECRET"),
"grant_type": "client_credentials"
}
response = requests.post(url, json=payload)
response.raise_for_status() # Raise an error for any bad responses
return response.json()["access_token"]
Key Consideration: Always handle authentication securely by using environment variables for storing sensitive information, and ensure that any errors (such as network failures or invalid credentials) are logged appropriately for troubleshooting.
4. Make API Requests
Once authenticated, you can begin interacting with Paycor’s API endpoints to fetch, create, or update data. The following function allows you to make GET, POST, PUT, or DELETE requests to various Paycor API endpoints.
def make_api_request(endpoint, method="GET", data=None):
base_url = "<https://api.paycor.com/v1>"
headers = {
"Authorization": f"Bearer {get_access_token()}",
"Content-Type": "application/json"
}
url = f"{base_url}/{endpoint}"
response = requests.request(method, url, headers=headers, json=data)
response.raise_for_status() # Raise an error for bad responses
return response.json()
Best Practice: Abstract the API request logic into reusable functions to simplify the integration process and make the codebase easier to maintain. For example, handling different request methods (GET, POST, etc.) with one function reduces repetitive code.
5. Example Usage
To fetch employee data, call the make_api_request
function and pass the relevant endpoint:
employees = make_api_request("employees")
print(employees)
This call retrieves employee information and prints it in JSON format.
Pro Tip: When dealing with large datasets (e.g., employee records), use pagination and filtering parameters to retrieve data in manageable chunks, which improves performance and reduces the risk of timeouts.
Notes
- Rate Limits: Paycor’s API enforces rate limits to ensure fair usage. Be sure to monitor the response headers that indicate your current rate limit status and handle
429 Too Many Requests
errors using an exponential backoff strategy. - Error Handling: Implement robust error handling for network failures, invalid requests, and expired tokens. Logging these errors will help with debugging and ensure smooth operation of your application.
Method 2: Building a Custom ETL Pipeline
Overview:
Building a custom ETL (Extract, Transform, Load) pipeline allows you to extract data from Paycor, transform it to meet your business needs, and load it into your own data warehouse. This method is suitable for applications that require scheduled synchronization of large datasets, such as payroll or employee records, for reporting and analysis.
Step-by-Step Guide
1. Understand Paycor API Documentation
Start by familiarizing yourself with the Paycor API documentation.
Identify the specific API endpoints you’ll be interacting with, such as those related to employee data, payroll, or time-off requests. Understanding the structure and data types of the responses will help you design your ETL pipeline more effectively.
2. Set Up Your Development Environment
As in Method 1, ensure that your environment is set up with the necessary libraries (requests
, python-dotenv
) and your API credentials are securely stored in a .env
file.
3. Extract Data from Paycor
The first phase of the ETL process is data extraction. Use the make_api_request
function to fetch the required data from Paycor.
def extract_employee_data():
return make_api_request("employees")
Best Practice: Consider implementing a batch extraction process for large datasets, where you paginate through the API results to avoid overloading your system or hitting rate limits.
4. Transform Data
Once the raw data is extracted, it must be transformed to fit the schema of your target database.
This could involve renaming fields, filtering out unnecessary data, or performing data type conversions.
def transform_employee_data(raw_data):
transformed_data = []
for employee in raw_data:
transformed_data.append({
"id": employee["id"],
"name": employee["name"],
"email": employee["email"],
# Add more fields as necessary
})
return transformed_data
Key Consideration: Ensure that your transformation logic is flexible enough to handle any changes in the API response format, such as newly introduced fields or data structures.
5. Load Data into Your Data Warehouse
The final step is loading the transformed data into your data warehouse.
Use an appropriate database connector (such as psycopg2
for PostgreSQL) to insert or update the records.
import psycopg2
def load_data_to_db(transformed_data):
connection = psycopg2.connect(
dbname=os.getenv("DB_NAME"),
user=os.getenv("DB_USER"),
password=os.getenv("DB_PASSWORD"),
host=os.getenv("DB_HOST")
)
cursor = connection.cursor()
for record in transformed_data:
cursor.execute("""
INSERT INTO employees (id, name, email) VALUES (%s, %s, %s)
ON CONFLICT (id) DO UPDATE SET name = EXCLUDED.name, email = EXCLUDED.email;
""", (record["id"], record["name"], record["email"]))
connection.commit()
cursor.close()
connection.close()
Pro Tip: Use ON CONFLICT clauses in your SQL queries to handle upserts (update or insert), ensuring that existing records are updated without creating duplicates.
Notes
- Scheduling: Automate this ETL process using scheduling tools like cron (Linux) or Airflow to run the pipeline at regular intervals. This ensures your data warehouse stays up to date with Paycor data.
- Monitoring and Logging: Monitor the performance of each phase (extraction, transformation, loading) and implement logging to capture errors. This will help maintain data accuracy and ensure smooth operation.
Method 3: Integrating with Paycor Using Bindbee
Overview - Bindbee is a unified API platform that lets you integrate with Paycor (and 50+ HRIS, ATS & Payroll Platforms) in just a few minutes. No-code, zero-maintenance integrations that offers round-the-clock data sync at one-tenth the cost of custom Integrations. Check out Bindbee today!
Setting up Paycor Integration with Bindbee
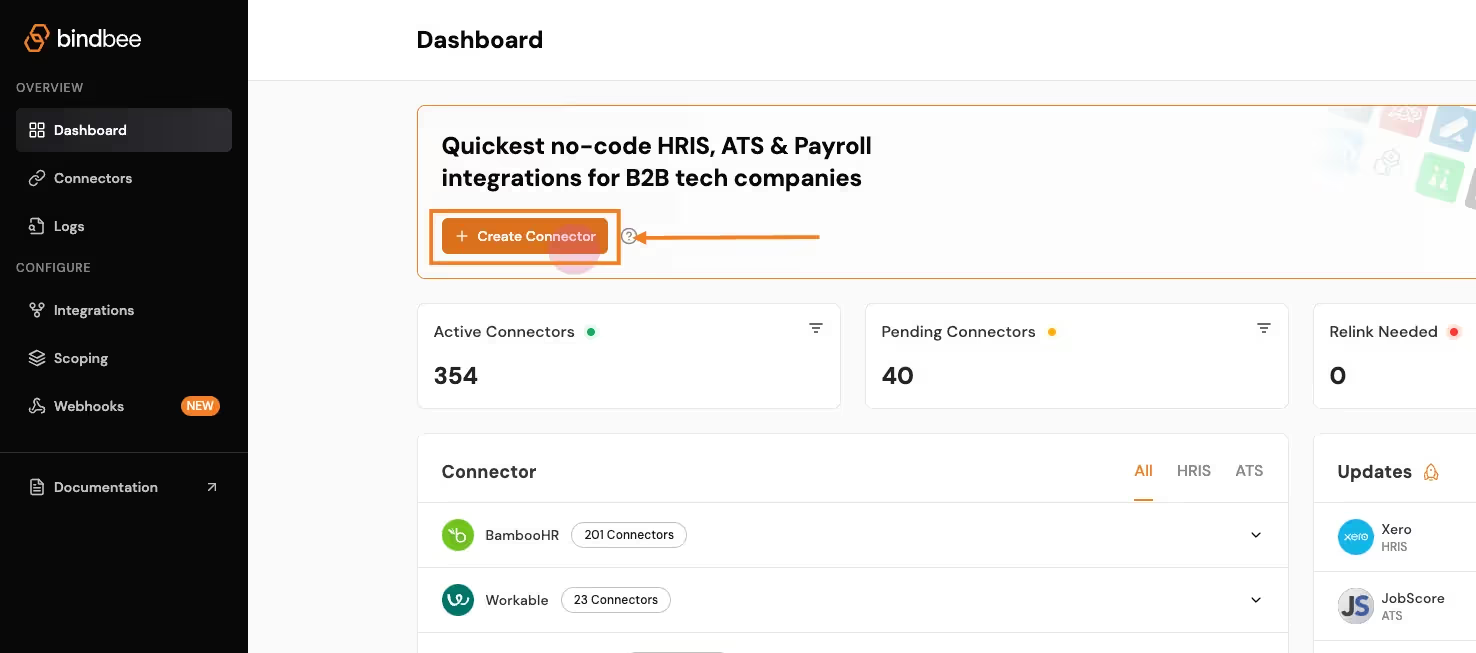
- Create a Connector:
- Click on Create Connector from the dashboard.
- Select HRIS as the type of integration. Enter customer details and give your connector a unique ID (e.g., Paycor_Integration).
- Generate a Magic Link:
- After setting up the connector, click Create Link to generate a magic link. This will allow the customer to authenticate the connection with Paycor API.
- Open the link and enter the necessary credentials (e.g., Paycor key, subdomain). This step establishes the connection between the platform and Bindbee.
- Sync the Connector:
- Once the connection is made, the connector will begin syncing data from Paycor. This may take a few minutes depending on the size of the data. You can track the sync status in the connector section.
- Access the Synced Data:
- After syncing, go to the Employee section in the Bindbee dashboard and select Get Employees to retrieve employee data from Paycor.
- Get the API Key and Connector Token:
- Copy the API key and the x-connector-token from the Bindbee dashboard, which you will need to make authenticated API requests.
Retrieving Employee Data with Bindbee
Once the Paycor data has been synced, you can retrieve employee data on to your application via the Bindbee API.
Here’s a step-by-step process for accessing synced data from Paycor through Bindbee’s unified API:
- Request Setup:
- Use the Bindbee API to send a request for employee data. You’ll need both your Bindbee API token and the x-connector-token.
- Example Request:
- Using Python:
import requests
url = "https://api.bindbee.com/hris/v1/employees"
headers = {
"Authorization": "Bearer YOUR_BINDBEE_API_KEY",
"x-connector-token": "YOUR_CONNECTOR_TOKEN",
"Accept": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
- Using cURL:
curl --request GET \
--url https://api.bindbee.com/hris/v1/employees \
--header 'Authorization: Bearer YOUR_BINDBEE_API_KEY' \
--header 'x-connector-token: YOUR_CONNECTOR_TOKEN'
This request will return a list of employee objects, including details like the employee’s first name, last name, job title, department, and contact information.
Sample Response:
{
"items": [
{
"id": "018b18ef-c487-703c-afd9-0ca478ccd9d6",
"first_name": "John",
"last_name": "Doe",
"job_title": "Chief Technology Officer",
"department": "Engineering",
"work_email": "john@johndoe.dev"
}
]
}
Bulk Employee Data Retrieval
For retrieving large datasets, Bindbee simplifies the process by allowing you to fetch bulk employee data from Sage.
The pagination feature helps manage large responses by returning results in pages.
What is Pagination?
“Pagination is the process of dividing a large dataset into smaller, manageable chunks or pages, allowing users to navigate through the data more easily without overwhelming them with too much information at once.”
- Pagination Parameters:
- Use the
cursor
andpage_size
parameters to navigate through the results. By default, Bindbee returns 50 records per page. - Example of a paginated request:
- Use the
url = "https://api.bindbee.com/hris/v1/employees?cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1&page_size=50"
response = requests.get(url, headers=headers)
- Querying for Specific Employee Data:
- You can further refine your request by filtering based on specific fields, such as manager_id, remote_id, or company_id to get employees under a particular manager or company.
Check out the entire documentation here - Bindbee’s API documentation.
Get Started with Paycor API Using Bindbee
Integrating with Paycor shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink—draining valuable engineering resources.

Let us handle the heavy lifting. You focus on growth. What say?
Book a demo with our experts today.