What is Paycom?
Paycom is an human capital management (HCM) solution designed to streamline payroll, human resources (HR), talent management, and employee engagement.
In this guide, we’ll explore how to integrate Paycom API into your applications, look at common use cases, and offer best practices for troubleshooting API issues. Whether you’re a developer, HR-tech professional, or part of a tech team evaluating Paycom, this blog aims to help you leverage the API effectively.
Note - This is an educational article on Paycom API. If you’re looking for an easier way to launch integrations with Paycom, check out Bindbee.
Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
That said, Here’s what we’ll cover in this article:
- A step-by-step Paycom guide using Direct API Integration and Bindbee.
- An overview of the API’s capabilities and key features.
- Best practices and troubleshooting tips for smooth API operations.
Understanding Paycom API
What is PaycomAPI?
The Paycom API is a robust RESTful service that allows developers to integrate Paycom's HR, payroll, and employee management functionalities into custom applications.
It supports secure data interactions, enabling easier access to employee records, time and attendance, payroll data, and benefits.
PaycomAPI - Key Use Cases
- Employee Management: Access and modify employee records, including personal details and job roles.
- Time and Attendance: Manage time-off requests, clock-ins/outs, and attendance records.
- Scheduling: Create and manage employee schedules efficiently.
- Payroll Processing: Integrate payroll data for accurate compensation calculations.
- Third-party Integrations: Integrate Paycom with LMS platforms to synchronize training records and employee progress.
Paycom API Integration Guide
We’ll walk through two main methodologies: Direct API Integration and using Binbdee.
Whether you’re comfortable with code or prefers to outsource the end-to-end integration process to a unified API like Bindbee, this section has you covered.
1. Direct API Integration
Overview: Direct API integration allows developers to interact directly with Paycom’s API by sending HTTP requests to specified endpoints.
Key Paycom API Endpoints
Below given are some of the most commonly used Paycom API endpoints for HR, payroll, and employee management.
This method provides maximum control over how the API is used but requires a solid understanding of authentication, error handling, and rate limiting.
PAYCOM API Integration - Step-by-Step Breakdown
1. Authentication
Paycom uses API SID and Token authentication. These credentials are provided by your Paycom representative and are required for securely accessing the API.
- OAuth 2.0: In most cases, you’ll authenticate using OAuth 2.0, which generates access tokens based on the user’s permissions.
- API Keys: Paycom may use API SID and Token, which must be passed in the request headers to authenticate.
Example for generating an access token using OAuth 2.0:
curl -X POST <https://api.paycom.com/oauth/token> \\
-H 'appkey: YOUR_APP_KEY' \\
-H 'Content-Type: application/x-www-form-urlencoded' \\
-d 'username=YOUR_USERNAME&password=YOUR_PASSWORD&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&grant_type=password'
“Note: The specific endpoints and parameters may vary based on your Paycom implementation. Always refer to Paycom's API documentation for the correct URLs and authentication methods.”
2. Making API Calls
After successfully authenticating, you can make HTTP requests such as GET, POST, PUT, DELETE to interact with Paycom’s data.
Below are examples for retrieving employee data and submitting time entries.
Example 1: Retrieve Employee Data
Here’s a basic example of how to retrieve employee data from the Paycom API using Python:
import requests
url = "<https://api.paycom.com/v1/employees>"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
This will return employee details, such as their first name, last name, and other relevant fields. You can easily modify the fields to include more detailed information, such as their job title or department.
Example 2: Retrieve Bulk Employee Data (Custom Reports)
If you need to retrieve data for multiple employees, you can use the custom report endpoint to generate bulk data.
url = "<https://api.paycom.com/v1/reports/custom>"
payload = {
"fields": ["firstName", "lastName", "jobTitle", "department"]
}
response = requests.post(url, json=payload, headers=headers)
print(response.json())
This will retrieve bulk data for employees based on the fields specified. The data returned is in JSON format, which can be parsed and integrated into your application as needed.
3. Error Handling and Response Parsing
Handling errors effectively is essential for maintaining robust integrations. Paycom’s API will return standard HTTP status codes (e.g., 200 OK, 400 Bad Request, 500 Internal Server Error), which must be managed in your code.
Example for handling responses and errors in Python:
if response.status_code == 200:
employee_data = response.json()
print("Employee Data:", employee_data)
else:
print(f"Error: {response.status_code} - {response.text}")
By implementing proper error handling, you can ensure that the application behaves predictably even when the API returns an error.
2. Data Extraction and Importation
Overview: Paycom’s API allows you to extract employee and payroll data efficiently and import external data into the system.
Steps for Data Extraction
- To retrieve employee or time data, make requests to endpoints such as
/api/v1/employees
or/api/v1/timeandattendance
. - You can loop through employee IDs to pull specific employee data or request custom reports for bulk extraction.
Example for extracting time and attendance data:
url = "<https://api.paycom.com/v1/timeandattendance>"
response = requests.get(url, headers=headers)
print(response.json())
Steps for Data Importation
- To import data such as time entries, use the relevant POST endpoint.
- Example for submitting a time entry:
time_entry = {
"employee_id": 123,
"start_time": "2024-06-01T09:00:00Z",
"end_time": "2024-06-01T17:00:00Z"
}
response = requests.post('<https://api.paycom.com/v1/timeandattendance>', json=time_entry, headers=headers)
3. Rate Limiting and Optimization
Overview: Paycom imposes rate limits to prevent overuse of the API.
To avoid exceeding these limits, developers need to implement strategies for throttling requests and managing rate limits effectively.
Steps to Manage Rate Limiting
- Implement a Rate Limiter that controls the number of API calls made per minute. This ensures that you stay within Paycom’s rate limits and avoid hitting errors like 429 Too Many Requests.
Example implementation of a rate limiter in Ruby:
class RateLimiter
def initialize(max_requests, period)
@max_requests = max_requests
@period = period
@request_count = 0
@start_time = Time.now
end
def throttle
if @request_count < @max_requests || Time.now - @start_time > @period
@request_count = 0 if Time.now - @start_time > @period
@start_time = Time.now if @request_count == 0
@request_count += 1
return true
else
return false
end
end
end
limiter = RateLimiter.new(100, 60) # 100 requests per minute
if limiter.throttle
# Make API call
else
# Wait and retry
end
4. Testing and Security Considerations
Testing:
To ensure your Paycom integration is working correctly, create unit tests for each API interaction. Use testing libraries such as RSpec (Ruby) or pytest (Python) to mock API responses and verify that your code behaves as expected.
Example: Using RSpec to test API responses in Ruby:
RSpec.describe PaycomClient do
it 'fetches employee data successfully' do
stub_request(:get, "<https://api.paycom.com/v1/employees>")
.to_return(status: 200, body: '{"id": 123, "name": "John Doe"}')
client = PaycomClient.new
response = client.get('/api/v1/employees')
expect(response.code).to eq(200)
expect(response.body).to include('John Doe')
end
end
Integrating with Paycom Using Bindbee
Overview - Bindbee is a unified API platform that lets you integrate with Paycom (and 50+ HRIS, ATS & Payroll Platforms) in just a few minutes. No-code, zero-maintenance integrations that offers round-the-clock data sync at one-tenth the cost of custom Integrations. Check out Bindbee today!
Setting up Paycom Integration with Bindbee
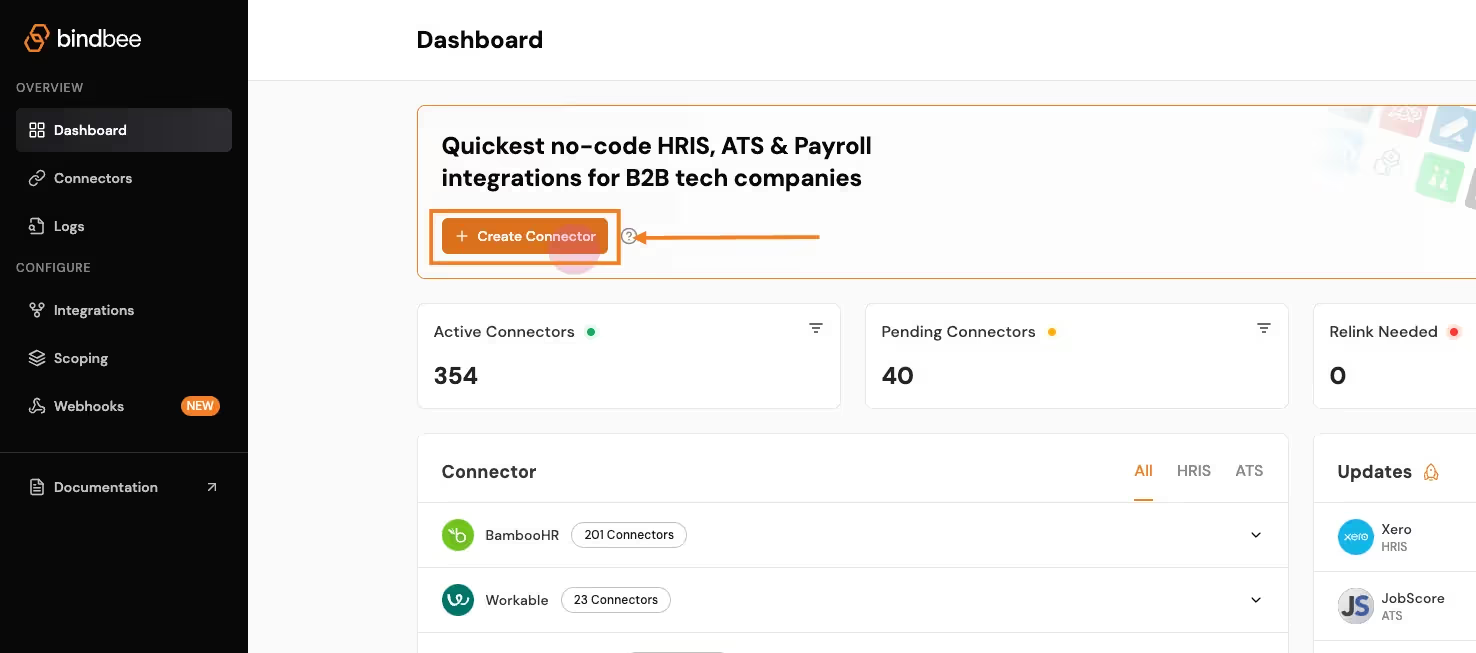
- Create a Connector:
- Click on Create Connector from the dashboard.
- Select HRIS as the type of integration. Enter customer details and give your connector a unique ID (e.g., Paycom_Integration).
- Generate a Magic Link:
- After setting up the connector, click Create Link to generate a magic link. This will allow the customer to authenticate the connection with UKG.
- Open the link and enter the necessary credentials (e.g., PaycomAPI key, subdomain). This step establishes the connection between Paycom and Bindbee.
- Sync the Connector:
- Once the connection is made, the connector will begin syncing data from Paycom. This may take a few minutes depending on the size of the data. You can track the sync status in the connector section.
- Access the Synced Data:
- After syncing, go to the Employee section in the Bindbee dashboard and select Get Employees to retrieve employee data from Paycom.
- Get the API Key and Connector Token:
- Copy the API key and the x-connector-token from the Bindbee dashboard, which you will need to make authenticated API requests.
Retrieving Employee Data with Bindbee
Once the Paycom data has been synced, you can retrieve employee data on to your application via the Bindbee API.
Here’s a step-by-step process for accessing synced data from Paycom through Bindbee’s unified API:
- Request Setup:
- Use the Bindbee API to send a request for employee data. You’ll need both your Bindbee API token and the x-connector-token.
- Example Request:
- Using Python:
import requests
url = "https://api.bindbee.com/hris/v1/employees"
headers = {
"Authorization": "Bearer YOUR_BINDBEE_API_KEY",
"x-connector-token": "YOUR_CONNECTOR_TOKEN",
"Accept": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
- Using cURL:
curl --request GET \
--url https://api.bindbee.com/hris/v1/employees \
--header 'Authorization: Bearer YOUR_BINDBEE_API_KEY' \
--header 'x-connector-token: YOUR_CONNECTOR_TOKEN'
This request will return a list of employee objects, including details like the employee’s first name, last name, job title, department, and contact information.
Sample Response:
{
"items": [
{
"id": "018b18ef-c487-703c-afd9-0ca478ccd9d6",
"first_name": "John",
"last_name": "Doe",
"job_title": "Chief Technology Officer",
"department": "Engineering",
"work_email": "john@johndoe.dev"
}
]
}
Bulk Employee Data Retrieval
For retrieving large datasets, Bindbee simplifies the process by allowing you to fetch bulk employee data from UKG.
The pagination feature helps manage large responses by returning results in pages.
What is Pagination?
“Pagination is the process of dividing a large dataset into smaller, manageable chunks or pages, allowing users to navigate through the data more easily without overwhelming them with too much information at once.”
- Pagination Parameters:
- Use the
cursor
andpage_size
parameters to navigate through the results. By default, Bindbee returns 50 records per page. - Example of a paginated request:
- Use the
url = "https://api.bindbee.com/hris/v1/employees?cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1&page_size=50"
response = requests.get(url, headers=headers)
- Querying for Specific Employee Data:
- You can further refine your request by filtering based on specific fields, such as manager_id, remote_id, or company_id to get employees under a particular manager or company.
Check out the entire documentation here - Bindbee’s API documentation.
Debugging Paycom API Integration
Below are key debugging strategies and steps to follow:
Step-by-Step Debugging Techniques
1. Start with Logs
- Ensure that API request and response logs are in place. Every API interaction should be logged with timestamps, status codes, and error messages (if any).
- Example:
logger.info(f"Request: {url}, Status Code: {response.status_code}, Response: {response.text}")
2. Check Authentication
- A common cause of API failures is incorrect authentication. Ensure that OAuth 2.0 tokens or API SID/Token are valid and included in the request header.
- If using OAuth 2.0, ensure the access token has not expired and is refreshed periodically.
- Debugging tip: If you receive an HTTP 401 Unauthorized error, verify that the correct credentials are being passed.
3. Handling HTTP Status Codes
Example for handling and logging HTTP errors:
if response.status_code == 200:
print("Success:", response.json())
elif response.status_code == 401:
logger.error("Authentication Error: Invalid API token.")
elif response.status_code == 429:
logger.warning("Rate Limit Exceeded. Retrying after a delay.")
else:
logger.error(f"API Error: {response.status_code}, {response.text}")
4. Use Postman or cURL for API Testing:
- Before integrating an API call into your codebase, use Postman or cURL to test endpoints and ensure they return the correct responses.
- Example using cURL:
curl -X GET <https://api.paycom.com/v1/employees> \\
-H "Authorization: Bearer YOUR_ACCESS_TOKEN"
5. Isolate the Issue
When an error occurs, narrow down the issue by isolating each part of the process.
For example, start by checking the authentication response, then move on to validating endpoint URLs, and finally inspect the payload or parameters.
Paycom API Integration - Best Practices
Authentication Best Practices
- Token Management:
- If using OAuth 2.0, implement automatic token refresh before the token expires. Monitor the expiration time of access tokens and refresh them proactively to avoid 401 Unauthorized errors.
- Store API keys and tokens securely using environment variables or a key management system—never hard-code credentials in your source code.
- Service Accounts:
- Use service accounts with minimal permissions to improve security. Assign roles and permissions that align with the specific API calls being made, ensuring no excessive access is granted.
API Request Optimization
- Efficient Data Retrieval:
- For endpoints that retrieve large datasets (e.g., employee or payroll data), implement pagination to handle responses in chunks and avoid hitting memory limits.
- Example: Use the
limit
andoffset
parameters in the Paycom API to paginate through large datasets:
url = "<https://api.paycom.com/v1/employees?limit=100&offset=200>"
- Batch API Calls:
- If you need to send multiple API requests, use batch processing techniques instead of making individual requests for each resource. This reduces the number of API calls and improves overall performance.
- Caching Frequently Requested Data:
- Implement caching for data that doesn’t change frequently, such as employee directory information. Caching helps reduce redundant API calls and speeds up the integration process.
- Example using a simple cache with Python:
cache = {}
def get_employee_data(employee_id):
if employee_id in cache:
return cache[employee_id]
else:
response = requests.get(f"<https://api.paycom.com/v1/employees/{employee_id}>")
cache[employee_id] = response.json()
return cache[employee_id]
Rate Limiting and Throttling:
- Paycom enforces rate limits on API calls, so ensure you implement a throttling mechanism to avoid hitting the 429 Too Many Requests error.
- Use exponential backoff when retrying failed API requests due to rate limits. This involves increasing the delay between retries progressively.
Example of exponential backoff:
import time
def exponential_backoff(retry_count):
time.sleep(2 ** retry_count)
retry_count = 0
while retry_count < 5:
response = make_api_call()
if response.status_code == 429:
retry_count += 1
exponential_backoff(retry_count)
else:
break
Error Handling Best Practices
- Graceful Degradation:
- Design your application to handle errors gracefully. For example, if a particular API call fails, the application should log the error, notify relevant stakeholders, and attempt a retry if possible, without crashing the entire system.
- Fallback Strategies:
- If an API call fails due to service downtime, implement fallback strategies that allow your application to continue functioning with minimal disruption. For example, use cached data or local data stores if the API is temporarily unavailable.
Security Best Practices
- Secure Data Transmission:
- Always use HTTPS to ensure that API requests and responses are encrypted during transmission.
- Monitor API Usage:
- Regularly monitor your API usage to detect unusual activity, such as unauthorized access attempts or excessive API requests. Set up alerts to notify you when certain thresholds are met, such as high error rates or frequent 401 Unauthorized responses.
- Version Control:
- Paycom’s API may introduce new versions over time. Always stay updated on the latest API version and ensure that your integration is compatible with future releases.
Get Started with Paycom Using Bindbee
Integrating with Paycom shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink—draining valuable engineering resources.
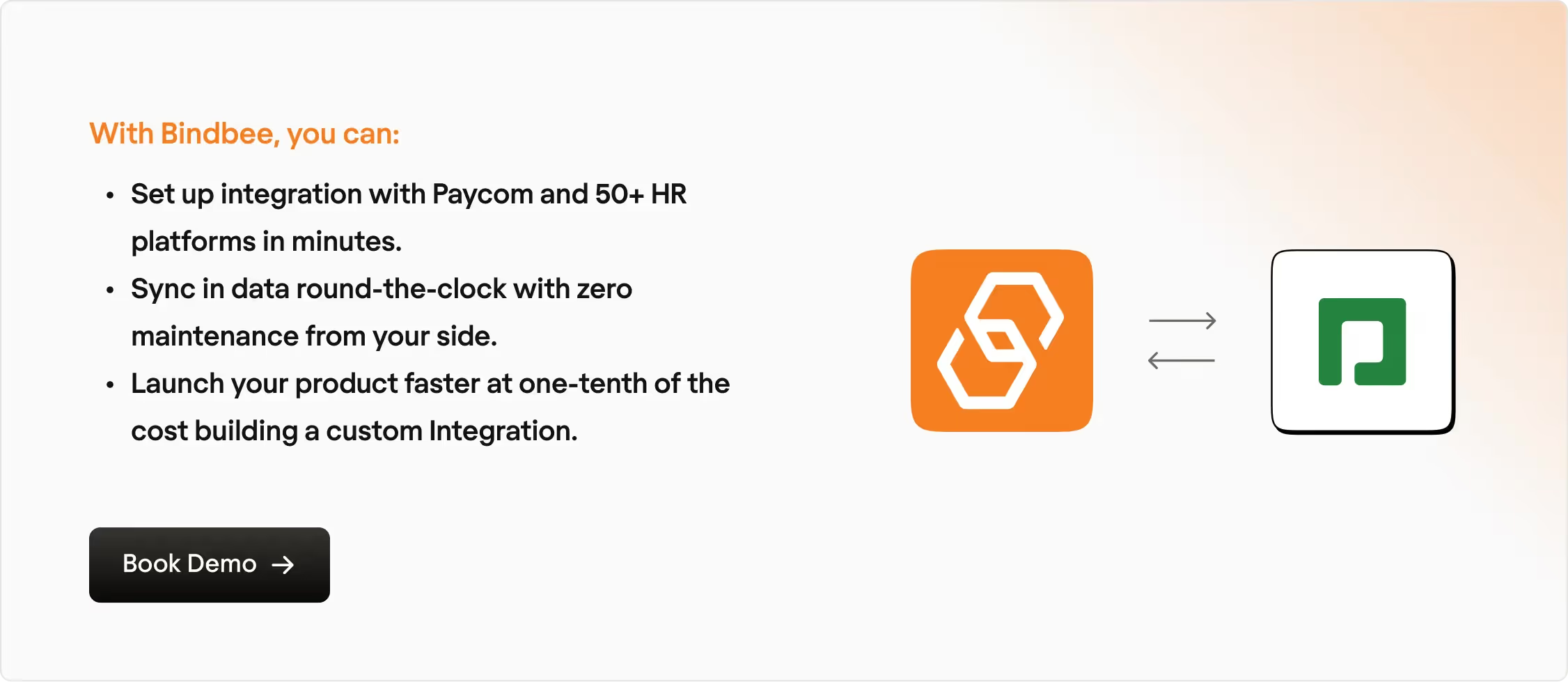
Let us handle the heavy lifting. You focus on growth, what say?
Book a demo with our experts today.