What is Namely?
Namely is a comprehensive HR software platform designed specifically for small to mid-sized businesses. It aims to simplify the complexities of human resources management, allowing organizations to focus on their people and culture.
In this guide, we’ll explore how to integrate Namely API into your applications, look at common use cases, and offer best practices for troubleshooting API issues. Whether you’re a developer, HR-tech professional, or part of a tech team evaluating Namely, this blog aims to help you leverage the API effectively.
Note - This is an educational article on Namely API. If you’re looking for an easier way to launch integrations with Namely, check out Bindbee.
Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
That said, Here’s what we’ll cover in this article:
- A step-by-step Namely API integration guide using Direct API and other key methodologies.
- An overview of the API’s capabilities and key features.
- Best practices and troubleshooting tips for easier API operations.
What is Namely API?
The Namely API is a RESTful API that allows developers to integrate and interact with the Namely HR platform, which manages various human resources functions such as payroll, benefits, and employee data.
Key Features of the Namely API
- REST-Based Architecture: The Namely API follows REST principles, meaning it utilizes standard HTTP methods (GET, POST, PUT, DELETE) for operations. Responses are typically in JSON format, making it easy to work with in web applications.
- Authentication: The API supports two primary authentication methods:
- OAuth 2.0: A standard for access delegation commonly used for token-based authentication.
- Bearer Token Authentication: A simpler method where a token is included in the request header.
- Data Access and Management: The Namely API provides various endpoints to manage HR-related data, including:
- Profiles Endpoint: Retrieve and manage employee profiles.
- Jobs Information Endpoint: View and update job-related details.
- Groups and Teams Endpoint: Organize employee profiles into groups and teams.
- Use Cases: The API can be utilized for various HR functions, such as:
- Onboarding new employees.
- Managing benefits and payroll data.
- Tracking time off and attendance.
- Conducting performance reviews and feedback.
Namely API Integration Guide
We’ll walk you through two key methodologies to integrate with Namely API.
Method 1: Direct API Integration
1. Prerequisites
Before starting the integration, make sure you have the following in place:
- Namely Account: Ensure you have an administrator-level Namely account to create access tokens and manage API integrations.
- API Documentation: Familiarize yourself with the Namely API documentation for an understanding of endpoint functionality, authentication methods, and data structures.
Pro Tip: Bookmark the API documentation for quick reference as you build and troubleshoot the integration.
2. Authentication
Namely provides two methods for authentication, allowing flexibility depending on the scope and security needs of your application.
1. Bearer Token Authentication
This is ideal for personal or small-scale integrations. You generate a token that can be included in every request.
- Steps to Generate a Personal Access Token:
- Log in to your Namely account as an administrator.
- Click on your profile icon and select API.
- Click New Access Token.
- Name your token for future reference and click Create.
- Copy and store this token securely; you will use it to authenticate API requests.
2. OAuth 2.0
OAuth 2.0 allows for more secure delegated access, useful for larger applications that require broader permissions or need to access multiple users' data.
For most third-party integrations, OAuth 2.0 ensures enhanced security.
Key Difference: While Bearer Token is simple to implement, OAuth 2.0 is more robust and scalable for complex applications.
3. Setting Up Your Development Environment
To start making API requests, set up your development environment with the necessary tools and libraries.
- HTTP Libraries: You’ll need libraries that allow you to make HTTP requests. For instance, if you’re working with Python, install the
requests
library to handle API calls.
pip install requests
- Postman: Consider using tools like Postman to test API endpoints before coding. Postman helps you validate requests and responses quickly.
4. Defining API Endpoints
Understanding key endpoints is essential for interacting with Namely’s resources. Below are commonly used endpoints for basic operations:
Notes
- Each endpoint can handle various HTTP methods (e.g., GET, POST, PUT, DELETE) depending on the operation you need to perform (retrieve, create, update, delete).
- The documentation for some categories (Home Feed, Profile Fields, Systems Info) can be accessed via the Namely API documentation for detailed information on how to use those endpoints.
5. Making API Requests
After setting up your environment, you can start making API requests.
Here’s how you can retrieve employee profiles from the Namely API using a Bearer Token:
import requests
# Set up your variables
sub_domain = 'your_sub_domain'
api_key = 'your_access_token'
url = f'https://{sub_domain}.namely.com/api/v1/profiles'
# Define headers
headers = {
'Accept': 'application/json',
'Authorization': f'Bearer {api_key}'
}
# Make the request
response = requests.get(url, headers=headers)
# Check response status
if response.status_code == 200:
profiles = response.json().get('profiles', [])
else:
print(f"Error: {response.status_code} - {response.text}")
Best Practice: Always include proper error handling and logging for each request to monitor the success and failure of API interactions.
6. Extracting Data
Once you’ve made successful requests, you’ll likely need to extract and process the data. Namely returns JSON data, so you’ll need to parse it to get the relevant fields.
Here’s how to extract specific information from the employee profiles:
employee_details = []
for profile in profiles:
first_name = profile.get('first_name')
last_name = profile.get('last_name')
dob = profile.get('dob')
employee_details.append({
'first_name': first_name,
'last_name': last_name,
'dob': dob
})
# Print or utilize the extracted data
for employee in employee_details:
print(f"First Name: {employee['first_name']}, Last Name: {employee['last_name']}, Date of Birth: {employee['dob']}")
Pro Tip: If the data set is large, implement pagination or filters to retrieve only the data you need. For example, you can limit requests to active employees or those in specific departments.
7. Handling Errors and Rate Limits
Namely API enforces rate limits to ensure fair usage, and errors can occur when those limits are exceeded or if requests are improperly formatted.
- Common HTTP Status Codes:
401 Unauthorized
: Incorrect or expired token.404 Not Found
: Invalid endpoint or resource.429 Too Many Requests
: Rate limits exceeded.
- Handling Rate Limits: If you receive a
429 Too Many Requests
error, implement a retry mechanism with exponential backoff to avoid overwhelming the API.
Example: Retry Logic for Rate Limit Errors:
import time
def make_request_with_retry(url, headers, max_retries=5):
retries = 0
while retries < max_retries:
response = requests.get(url, headers=headers)
if response.status_code == 429: # Too many requests
wait_time = int(response.headers.get('Retry-After', 1))
time.sleep(wait_time)
retries += 1
else:
return response
return None
8. Testing and Validation
Validate that the following aspects work as expected:
- Endpoints: Ensure you can access all necessary endpoints and that they return correct data.
- Data Synchronization: Verify that data is correctly fetched, updated, or deleted as per your application's requirements.
- Error Handling: Test various error scenarios (e.g., invalid tokens, rate limit exceedances) to confirm your error handling logic works correctly.
Method 2: Integrating with Namely Using Bindbee
Overview - Bindbee is a unified API platform that lets you integrate with Namely (and 50+ HRIS, ATS & Payroll Platforms) in just a few minutes. No-code, zero-maintenance integrations that offers round-the-clock data sync at one-tenth the cost of custom Integrations. Check out Bindbee today!
Setting up Namely Integration with Bindbee
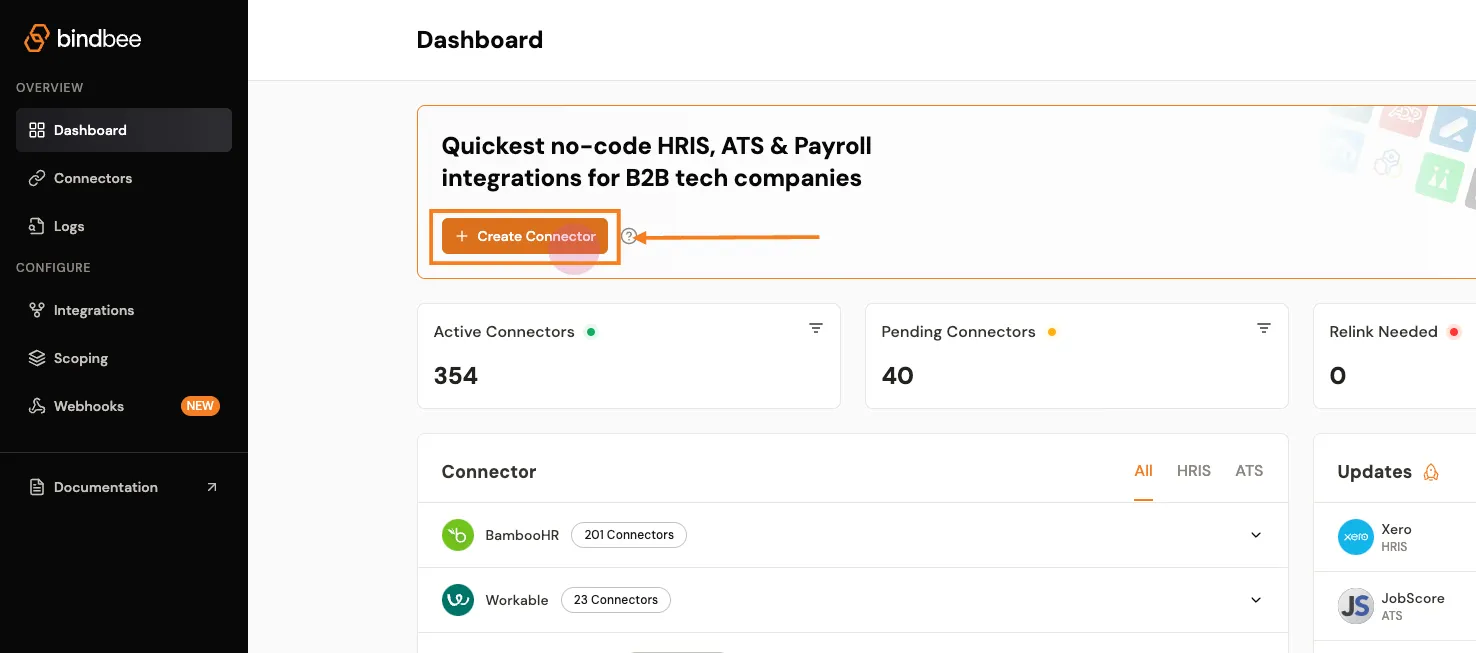
- Create a Connector:
- Click on Create Connector from the dashboard.
- Select HRIS as the type of integration. Enter customer details and give your connector a unique ID (e.g., Namely_Integration).
- Generate a Magic Link:
- After setting up the connector, click Create Link to generate a magic link. This will allow the customer to authenticate the connection with Namely API.
- Open the link and enter the necessary credentials (e.g., Namely key, subdomain). This step establishes the connection between the platform and Bindbee.
- Sync the Connector:
- Once the connection is made, the connector will begin syncing data from Namely. This may take a few minutes depending on the size of the data. You can track the sync status in the connector section.
- Access the Synced Data:
- After syncing, go to the Employee section in the Bindbee dashboard and select Get Employees to retrieve employee data from Namely.
- Get the API Key and Connector Token:
- Copy the API key and the x-connector-token from the Bindbee dashboard, which you will need to make authenticated API requests.
Retrieving Employee Data with Bindbee
Once the Namely data has been synced, you can retrieve employee data on to your application via the Bindbee API.
Here’s a step-by-step process for accessing synced data from Namely through Bindbee’s unified API:
- Request Setup:
- Use the Bindbee API to send a request for employee data. You’ll need both your Bindbee API token and the x-connector-token.
- Example Request:
- Using Python:
import requests
url = "https://api.bindbee.com/hris/v1/employees"
headers = {
"Authorization": "Bearer YOUR_BINDBEE_API_KEY",
"x-connector-token": "YOUR_CONNECTOR_TOKEN",
"Accept": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
- Using cURL:
curl --request GET \
--url https://api.bindbee.com/hris/v1/employees \
--header 'Authorization: Bearer YOUR_BINDBEE_API_KEY' \
--header 'x-connector-token: YOUR_CONNECTOR_TOKEN'
This request will return a list of employee objects, including details like the employee’s first name, last name, job title, department, and contact information.
Sample Response:
{
"items": [
{
"id": "018b18ef-c487-703c-afd9-0ca478ccd9d6",
"first_name": "John",
"last_name": "Doe",
"job_title": "Chief Technology Officer",
"department": "Engineering",
"work_email": "john@johndoe.dev"
}
]
}
Bulk Employee Data Retrieval
For retrieving large datasets, Bindbee simplifies the process by allowing you to fetch bulk employee data from Sage.
The pagination feature helps manage large responses by returning results in pages.
What is Pagination?
“Pagination is the process of dividing a large dataset into smaller, manageable chunks or pages, allowing users to navigate through the data more easily without overwhelming them with too much information at once.”
- Pagination Parameters:
- Use the
cursor
andpage_size
parameters to navigate through the results. By default, Bindbee returns 50 records per page. - Example of a paginated request:
- Use the
url = "https://api.bindbee.com/hris/v1/employees?cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1&page_size=50"
response = requests.get(url, headers=headers)
- Querying for Specific Employee Data:
- You can further refine your request by filtering based on specific fields, such as manager_id, remote_id, or company_id to get employees under a particular manager or company.
Check out the entire documentation here - Bindbee’s API documentation.
Get Started with Namely API Using Bindbee
Integrating with Namely shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink—draining valuable engineering resources.
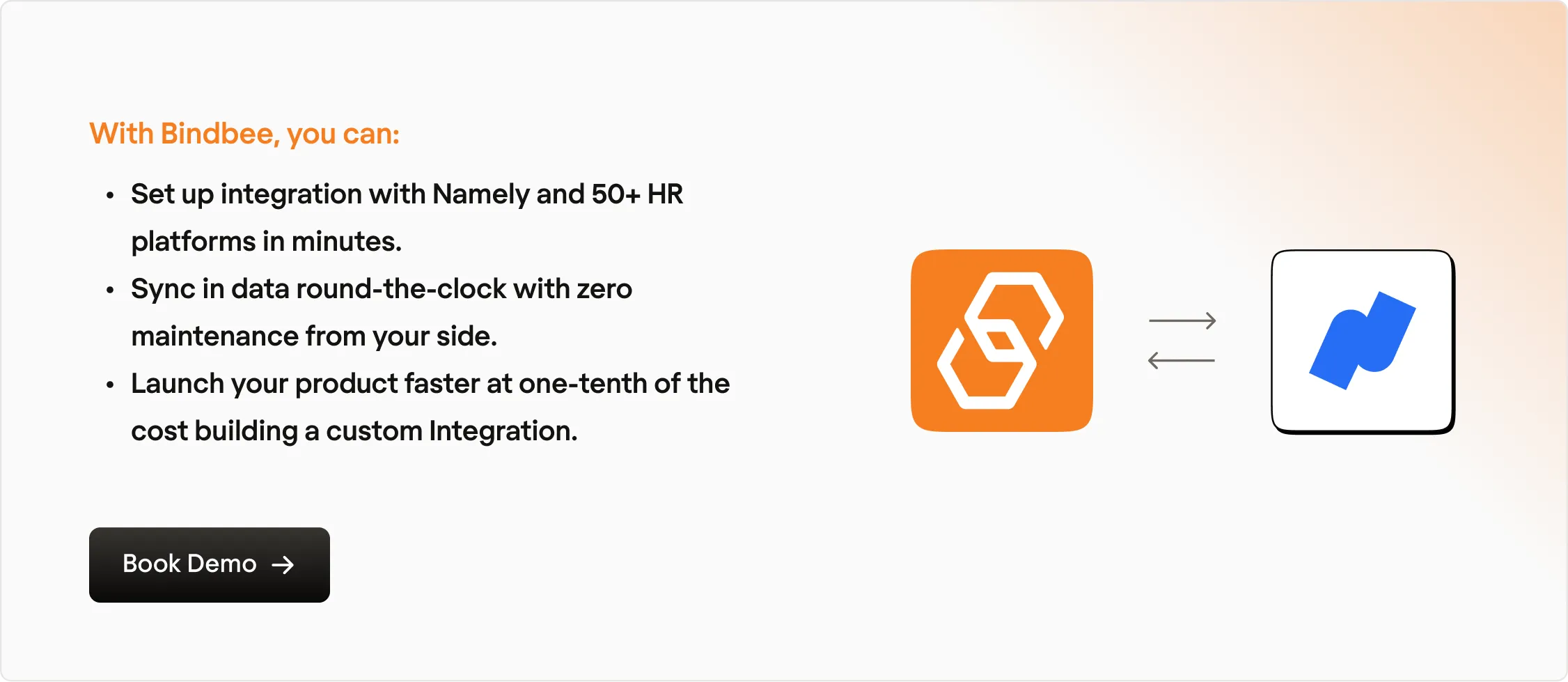
Let us handle the heavy lifting. You focus on growth, what say?
Book a demo with our experts today.