In today's fast-paced HR world, having your finger on the pulse of employee data isn't just nice—it's essential. Enter BambooHR, the cloud-based HR powerhouse that's changing the game with its nifty API. Whether you're an HR guru or a code wizard, this API is your ticket to pulling employee info like a pro. Buckle up, because we're about to dive into the nitty-gritty of tapping into the BambooHR API. We'll show you the ropes with real-world examples and code snippets that'll have you slinging HR data in no time.
Understanding the BambooHR API Ecosystem
Before diving into the technical details, let's explore the key components of the BambooHR API:
Base URL Structure
The BambooHR API follows a consistent URL pattern:
https://api.bamboohr.com/api/gateway.php/{subdomain}
Authentication Mechanism
BambooHR uses API keys for secure access, ensuring that only authorized users can retrieve sensitive employee data.
Response Formats
The API supports both JSON and XML response formats, allowing you to choose the one that best fits your integration needs.
Core Functionalities
- Employee data retrieval and updates
- Company file and report generation
- Time off and benefits management
- Time and goal tracking
- Training record access
Setting Up Your BambooHR API Access
Before you can start pulling employee data, you need to set up your API access:
- Log in to your BambooHR account
- Navigate to Settings > Account > API Keys
- Generate a new API key
Remember: Your API key inherits the permissions of the user who created it. Ensure you have the necessary access levels for the data you intend to retrieve.
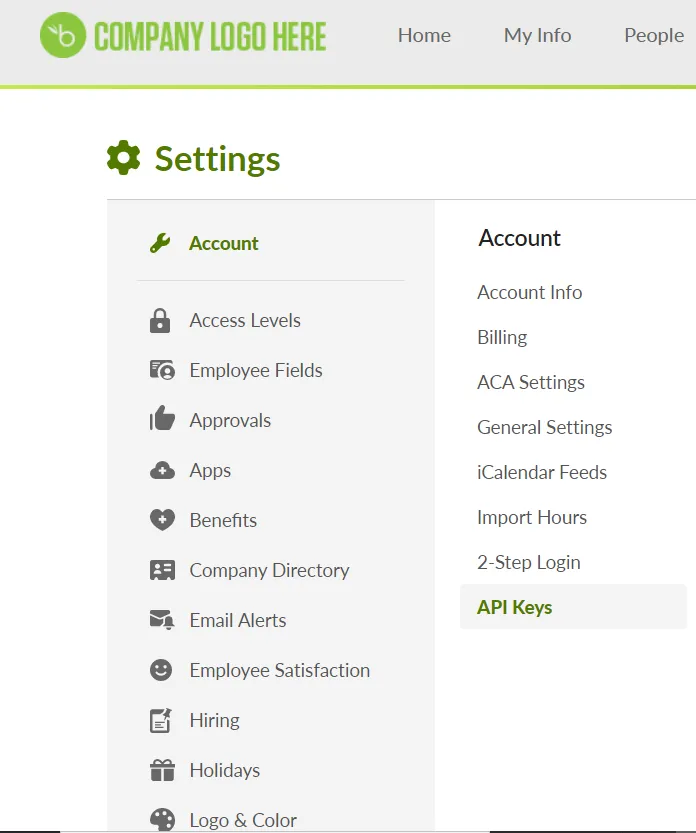
Three Powerful Approaches to Employee Data Retrieval
Let's explore three effective methods to pull employee data from the BambooHR API:
- Python Integration
- Postman API Testing
- Bindbee Unified API Platform
1. Python
Python's `requests` library provides a simple yet powerful way to interact with the BambooHR API. Here's a script to fetch data for a single employee:'
1mport requests
2
3# API endpoint
4url = "https://api.bamboohr.com/api/gateway.php/your_company_domain/v1/employees/4/?fields=firstName,lastName,age,gender,jobTitle,department,division"
5
6headers = {
7 "Accept": "application/json"
8}
9
10# Make the API call
11response = requests.get(url, headers=headers, auth=('your_api_key', 'any_string'))
12
13# Print the response
14print(response.json())
15```
16
17This script retrieves data for employee ID 4, including their name, age, gender, job title, department, and division.
18
19For bulk employee data retrieval, leverage BambooHR's custom reports endpoint:
20
21```python
22import requests
23
24url = "https://api.bamboohr.com/api/gateway.php/your_company_domain/v1/reports/custom?format=JSON"
25
26payload = {
27 "fields": ["firstName", "lastName", "age", "gender", "jobTitle", "department", "division"]
28}
29
30headers = {
31 "Content-Type": "application/json"
32}
33
34response = requests.post(url, json=payload, headers=headers, auth=('your_api_key', 'any_string'))
35
36print(response.json())
This script generates a custom report containing the specified fields for all employees.
2. Postman: Intuitive API Testing and Exploration
Postman is a tool designed for creating and utilizing APIs, offering a user-friendly interface for API interactions. You can retrieve employee data with Postman using the same details you used with Python, such as endpoints, API keys, and parameters.
To start, you can set up a new collection to organize your requests, which simplifies authentication when multiple requests share the same authorization settings.
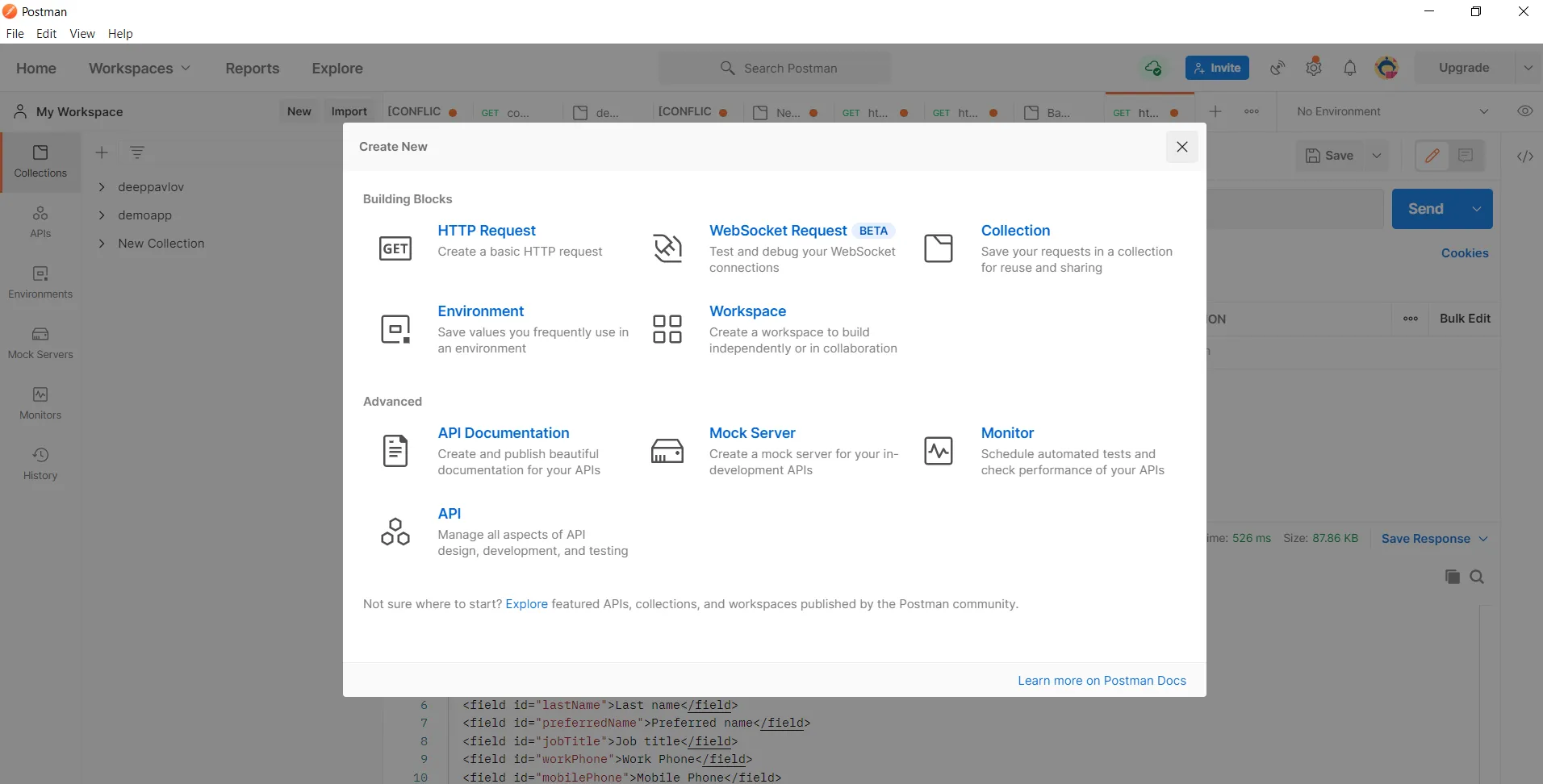
After creating your collection, go to the authorization section and choose the Basic Auth option. Enter your API key in the username field

“Add a new request to your collection. For your initial API call with Postman, you’ll retrieve data for a single employee. Under Authorization, choose the Basic Auth option, which will inherit the API key from your collection. Then, enter the API endpoint in the request URL field. The standard endpoint for this call is
https://api.bamboohr.com/api/gateway.php/{companyDomain}/v1/employees/{id}
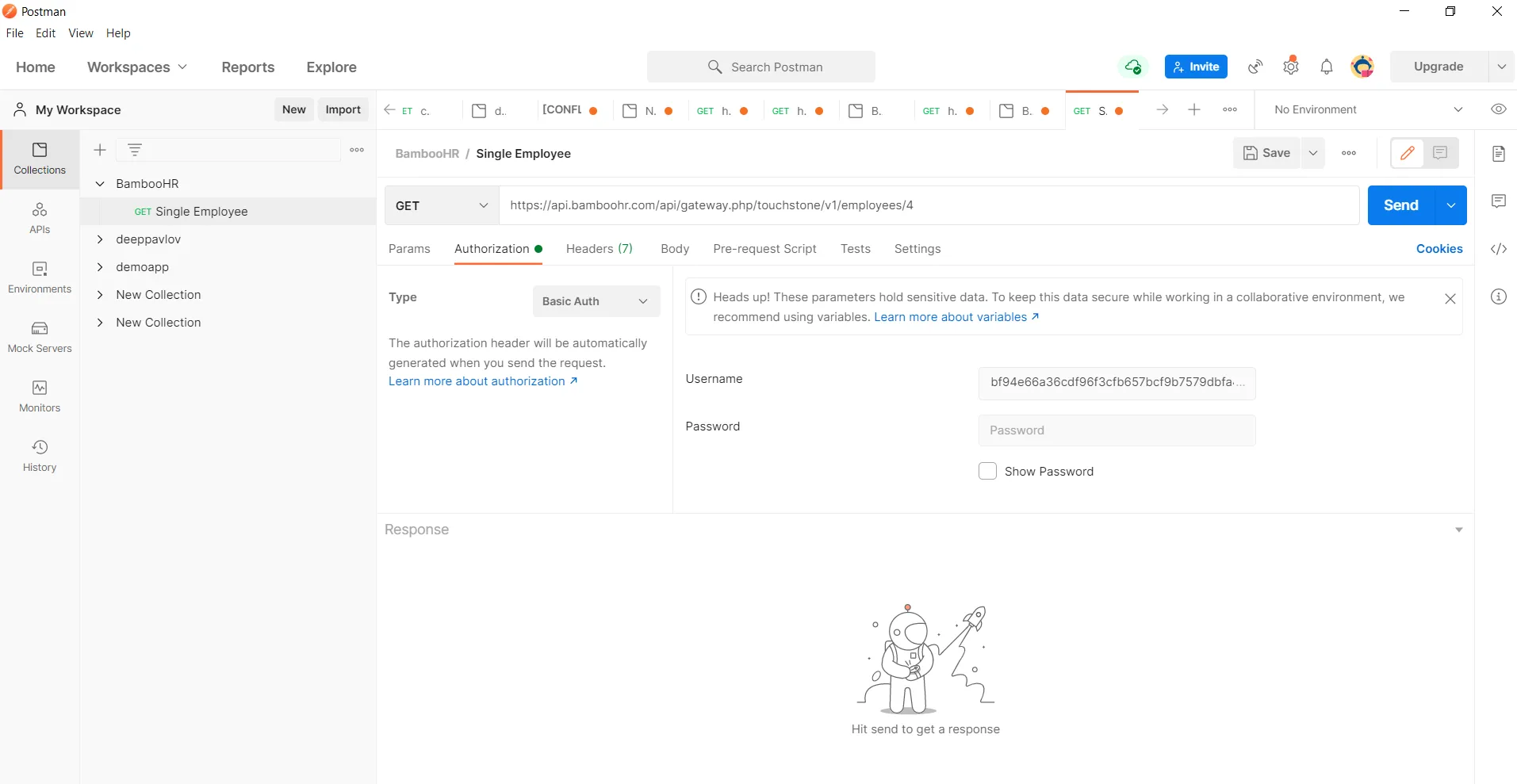
The final step for this call is to navigate to the Params tab and enter the required query parameters. Use ‘fields’ as the key and list all necessary field names, separated by commas, as the value. Sending this request should yield results similar to the following.

By default, the response is formatted in XML, but you can change this by editing the Accept parameter under the Headers tab. To change the format to JSON, replace the default value of application/xml with application/json.
You can follow similar steps to retrieve bulk employee data from BambooHR using custom reports in Postman.
Start by creating a new request within your collection, then choose the 'Basic Auth' option. Enter the required endpoint:
https://api.bamboohr.com/api/gateway.php/{companyDomain}/v1/reports/custom,
which uses the POST method instead of GET. Make sure to select POST from the dropdown.

Next, include the format variable in the Params tab

Include a Content-Type parameter in the Headers tab.

This will display results similar to the following.
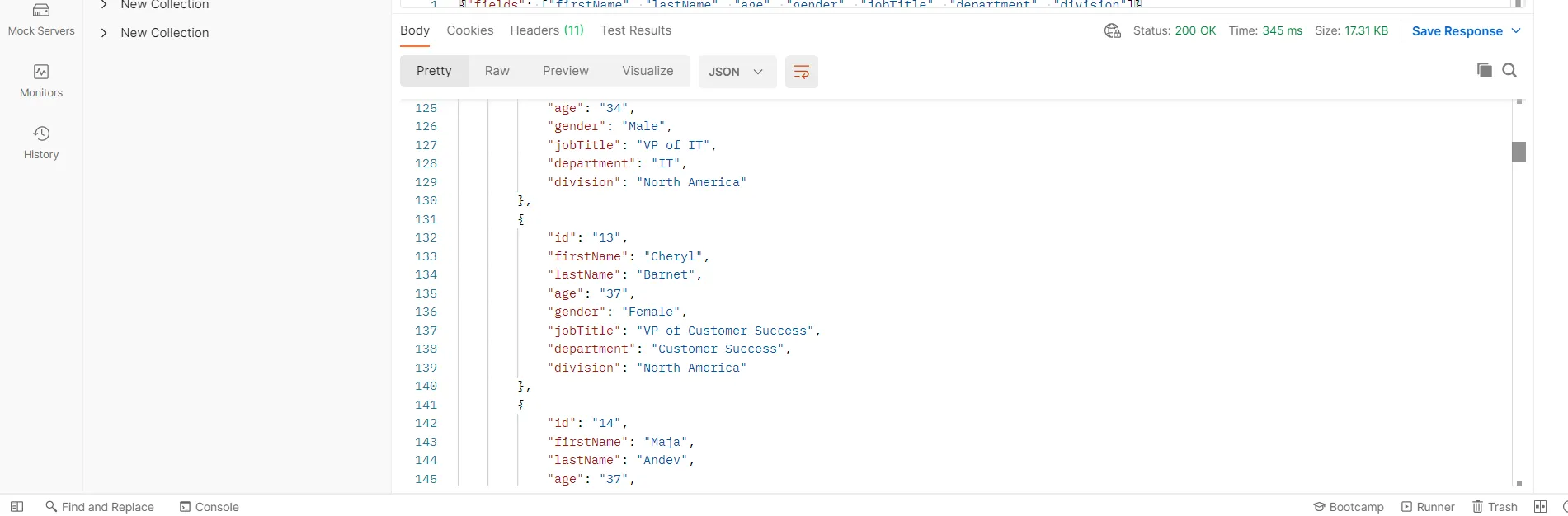
3. How to Retrieve Employees Using Bindbee
Now, let's talk about Bindbee—your secret weapon for API integrations. Bindbee simplifies the process of aggregating data from multiple sources, including BambooHR. Here's how to get started:
Sign in with your Bindbee credentials, If you don’t have credentials, book a demo from here and we will get in touch with you.
Once you have signed in, You will need to click on the Create Connector button and fill in all the details to create a Magic link. Bindbee’s Magic Link feature offers B2B tech companies an easy way to deliver an in-browser Link experience to their end-users without requiring any front-end code.

Once you have signed in, You will need to click on the Create Connector button and fill in all the details to create a Magic link. Bindbee’s Magic Link feature offers B2B tech companies an easy way to deliver an in-browser Link experience to their end-users without requiring any front-end code.

Then Select Bamboo HR from the list of HRIS

Now the magic link has been created.
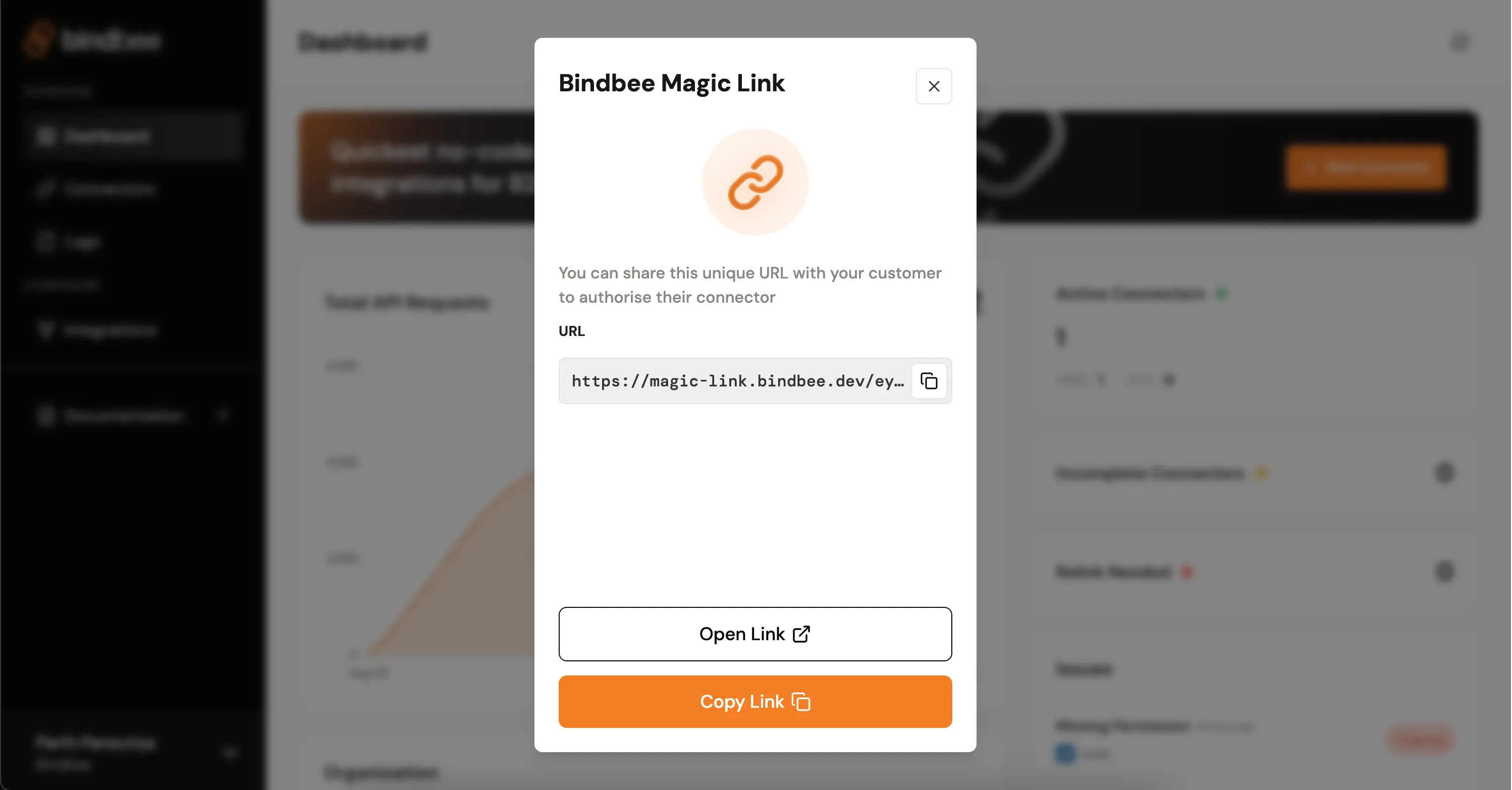
You need to open this link and authorize yourself.

And then the connections have been established:

You can follow this link for more detailed information.
After connecting if you want the data from bindbee follow this:
Authentication
Learn about the process of authenticating your requests to the Bindbee API.
Overview
When initiating requests to the Bindbee API, it is essential to include the necessary authentication parameters to establish your identity as an authorized user.
There are two primary authentication protocols:
- Bindbee API Key
- Connector Tokens
Credentials obtained through these protocols must be incorporated into the headers of each request sent to the Bindbee API.
Bindbee API Key
For any communication with the Bindbee API, it is crucial to have an API key to authenticate yourself as an authorized user.
It is recommended to securely store your access key after its creation in Bindbee
In case you no longer have access to your key, you have the option to regenerate you Bindbee API Key in API Key Tab in Settings.
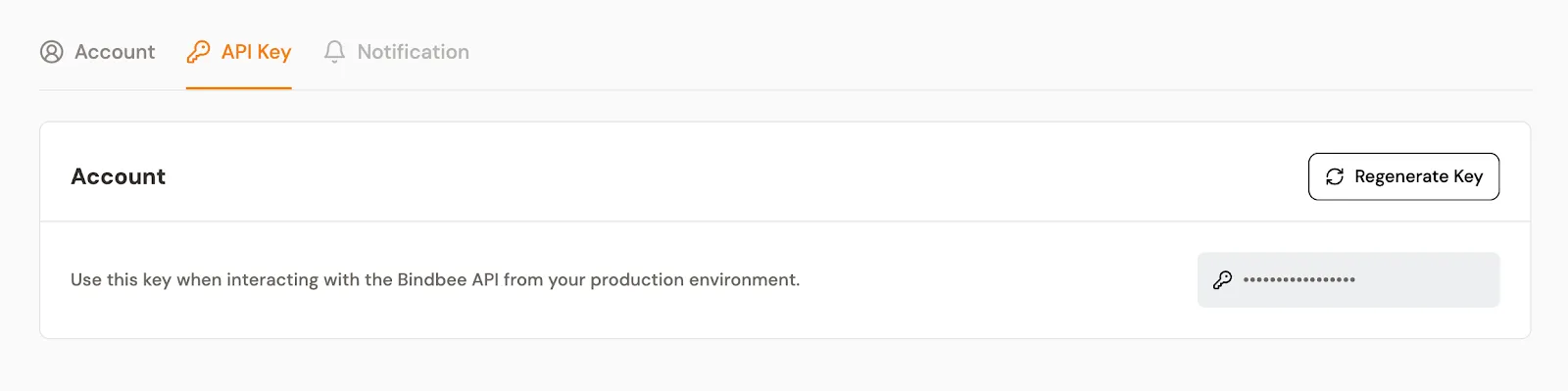
When creating your own requests, it is essential to include your API key with a “Bearer ” prefix as a header named Authorization
. This practice is crucial for authorizing your Bindbee API requests effectively. Ensure that this specific header is incorporated in every request, adhering to the following format:
Authorization:
Bearer YOUR_BINDBEE_API_KEY
Connector Tokens
When issuing requests to the Bindbee API concerning your end users’ data, access or manipulation, authorization is granted only if the users have undergone the Bindbee’s Magic Link process and successfully stored their connector_token
, as this token is a prerequisite for the successful execution of such requests.
You can find your connector_token
inside the Overview section of your connector

When creating your own requests, it is essential to include your user’s connector_token
as a header named X-Connector-Token
for authorizing your Bindbee API requests effectively. Ensure that this specific header is incorporated in every request, adhering to the following format:
X-Connector-Token: END_USER_CONNECTOR_TOKEN
Conclusion
In this discussion, we explored various methods to interact with BambooHR's API, including Python, Postman, and BindBee. We examined the workings of each approach and how they can be implemented.
BindBee offers a unified API platform that simplifies integration across multiple HRIS, ATS and Payroll platforms. It provides a centralized interface for efficiently managing your company's digital assets and information.
If you're interested in integrating your systems with popular HR and recruitment platforms like BambooHR, Workday or other leading HR tools, consider exploring our comprehensive guides on HRIS integrations.
To learn more about how BindBee can streamline your integration processes, you can book a demo to reach out to our integration specialists for a personalized demonstration.