What is What is Hibob?
Bob HR, formerly known as HiBob, is a cloud-based human resource management system (HRMS) designed to streamline HR processes for mid-sized and fast-growing companies. The platform offers a comprehensive suite of features that facilitate efficient employee management and experience.
In this guide, we’ll explore how to integrate Bob API into your applications, key features and look at common use cases of the Bob API. Whether you’re a developer, HR-tech professional, or part of a tech team evaluating Bob, this blog aims to help you leverage the API effectively.
Note - This is an educational article on Bob API. If you’re looking for an easier way to launch integrations with Bob, check out Bindbee.
Our Unified API connects you to 50+ HRIS, ATS and Payroll Platforms in minutes. Zero maintenance, no-code Integrations that lets you launch at one-tenth the cost of custom Integrations.
That said, Here’s what we’ll cover in this article:
- A step-by-step Bob API integration guide using Direct API and other key methodologies.
- An overview of the API’s capabilities and key features.
- Best practices and troubleshooting tips for easier API operations.
What is Bob API?
Bob API is a RESTful API designed to integrate and interact with Hibob's HRMS platform along with its HR functionalities
Key Features of the Bob API
Architecture
The Hibob API is based on REST architecture, which allows for stateless communication between clients and servers. This makes it straightforward to integrate with various applications and services.
Key Endpoints
Authentication
The API uses OAuth 2.0 for secure authentication, ensuring only authorized applications can access sensitive HR data. Each integration requires a unique service ID and token, which are generated during account registration.
Rate Limiting
To maintain stability and performance, the API enforces rate limits based on subscription tiers:
- Free accounts: Up to 100 requests per minute.
- Enterprise accounts: Up to 2,000 requests per minute.
Webhooks
Hibob supports webhooks, allowing real-time notifications for specific events. This feature keeps external systems synchronized with any changes in employee data.
SDKs
Official SDKs are available in several programming languages, including Node.js, PHP, .NET, and Python. These SDKs simplify integration by offering pre-built functions for common tasks.
Sandbox Environment
A sandbox environment is available for developers to test integrations safely, without affecting live data. This enables secure testing and debugging.
Use Cases
The Hibob API is ideal for organizations that want to:
- Automate HR workflows by integrating with systems such as payroll or performance management tools.
- Improve data visibility through real-time synchronization across platforms.
- Streamline onboarding and off-boarding processes by managing employee data programmatically.
Bob API Integration Guide
We’ll walk you through two key methodologies to integrate with Bob API.
Method 1 - Direct API Integration
1. Authentication Setup
Before you can make API calls, you need to authenticate your requests using OAuth 2.0.
This ensures secure access to Bob's data.
Steps to Create a Service User
- Navigate to Integrations:
- In Bob, go to Settings > Integrations.
- Manage Service Users:
- Click Manage Service Users and create a new user.
- After creation, you'll receive a Service User ID and a Token. These will be used to authenticate all API requests.
Example: Requesting Authentication
curl -X POST <https://app.hibob.com/api/login> \\
-H "Content-Type: application/json" \\
-d '{"user": "SERVICE_USER_ID", "token": "SERVICE_USER_TOKEN"}'
This cURL command sends a request to Bob's API to authenticate using the Service User ID and Token you generated.
2. API Access Configuration
After authentication, you need a tokenized URL and Secret Key to start using the API.
Steps to Generate a Tokenized URL
- Go to Settings in Bob, and under the Integration Setup section, generate a tokenized URL and a Secret Key.
- This tokenized URL allows your application to communicate securely with Bob.
Example Use Case: Connecting to a Payroll System
Consider integrating Bob with a payroll system.
You’d set up the integration by entering the tokenized URL and Secret Key into the payroll system’s integration interface. This allows the payroll system to pull employee data from Bob in real time.
Example: Connecting Using Tokenized URL
import requests
url = "<https://api.hibob.com/v1/employees>"
headers = {
"Authorization": "Bearer YOUR_SECRET_KEY"
}
response = requests.get(url, headers=headers)
print(response.json())
This Python example shows how to authenticate and retrieve employee data using the tokenized URL and secret key.
3. Data Mapping
Data mapping ensures that fields in your system correctly correspond to Bob's API fields. This is crucial for maintaining data integrity across platforms.
Steps for Data Mapping
- Identify Key Fields: Map Bob's fields (e.g.,
firstName
,email
,employeeId
) to your system. - Map Custom Fields: Use Bob’s Data Mapping tool to align your custom fields with Bob’s predefined fields.
Example Use Case: Onboarding New Employees
When onboarding new employees through an ATS, you would map the ATS fields like Candidate Name and Job Title to Bob's corresponding fields like firstName and position.
Example Mapping Code
{
"email": "employee@example.com",
"firstName": "Jane",
"lastName": "Doe",
"work": {
"title": "Software Engineer",
"department": "Engineering"
}
}
This JSON structure shows how data from an external system (e.g., ATS) can be mapped to Bob’s API to create new employee entries.
4. Interacting with API Endpoints
Bob provides a variety of RESTful API endpoints to interact with.
Below are some common API calls with detailed examples.
Key API Endpoints
Example: Retrieving Employee Data
curl -X GET <https://api.hibob.com/v1/employees> \\
-H "Authorization: Bearer YOUR_SECRET_KEY"
This request retrieves all employee data from Bob's API, which can be used to sync with other systems like payroll.
Example Use Case: Updating Employee Information
For example, if an employee's role changes, you can use the API to update their information in real-time:
curl -X PUT <https://api.hibob.com/v1/employees/{employeeId}> \\
-H "Content-Type: application/json" \\
-H "Authorization: Bearer YOUR_SECRET_KEY" \\
-d '{"work": {"title": "Lead Engineer", "department": "Engineering"}}'
5. Setting Up Webhooks for Real-Time Updates
Webhooks allow your application to stay in sync with Bob by receiving real-time notifications when events occur, such as employee status changes or new time-off requests.
Steps to Set Up Webhooks
- Subscribe to Webhook Events:
- Go to Settings > Webhooks in Bob and subscribe to events like
employee_created
,employee_updated
, ortime_off_requested
.
- Go to Settings > Webhooks in Bob and subscribe to events like
- Provide a Webhook URL:
- Specify a URL in your system where Bob will send POST requests when events happen.
Example Use Case: Time Off Requests
When an employee submits a time-off request, Bob will send a notification to your system's webhook, allowing your app to update its calendar or approval system in real-time.
Example: Webhook Handling
from flask import Flask, request
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def handle_webhook():
data = request.json
# Process the data (e.g., log it or update the system)
print(f"Received webhook: {data}")
return '', 200
if __name__ == '__main__':
app.run(port=5000)
This Python Flask app listens for incoming webhook events from Bob and processes the data accordingly.
6. Testing and Validation
Before going live, use Bob's sandbox environment to test your integration without affecting live data. This step allows you to experiment with data mapping, endpoint interactions, and webhook notifications safely.
Steps for Testing
- Test API Requests: Use the sandbox to simulate creating, updating, or deleting employees.
- Validate Webhooks: Ensure that your webhook listener processes incoming events correctly.
- Review Logs: Check your system and Bob’s logs to identify any discrepancies or errors.
Example: Testing API Calls in a Sandbox
curl -X POST <https://sandbox-api.hibob.com/v1/employees> \\
-H "Authorization: Bearer SANDBOX_SECRET_KEY" \\
-d '{"firstName": "John", "lastName": "Doe", "work": {"title": "QA Engineer"}}'
7. Deployment and Monitoring
After validating the integration in the sandbox, you can deploy the integration to the live environment.
Best Practices for Monitoring
- API Performance: Monitor API response times and error rates.
- Webhook Failures: Set up retry mechanisms in case a webhook fails to deliver.
- Logs: Use logging to track all API interactions and webhook events, ensuring any issues are quickly identified and resolved.
Method 3: Integrating with Bob Using Bindbee
Overview - Bindbee is a unified API platform that lets you integrate with Bob (and 50+ HRIS, ATS & Payroll Platforms) in just a few minutes. No-code, zero-maintenance integrations that offers round-the-clock data sync at one-tenth the cost of custom Integrations. Check out Bindbee today!
Setting up Bob Integration with Bindbee
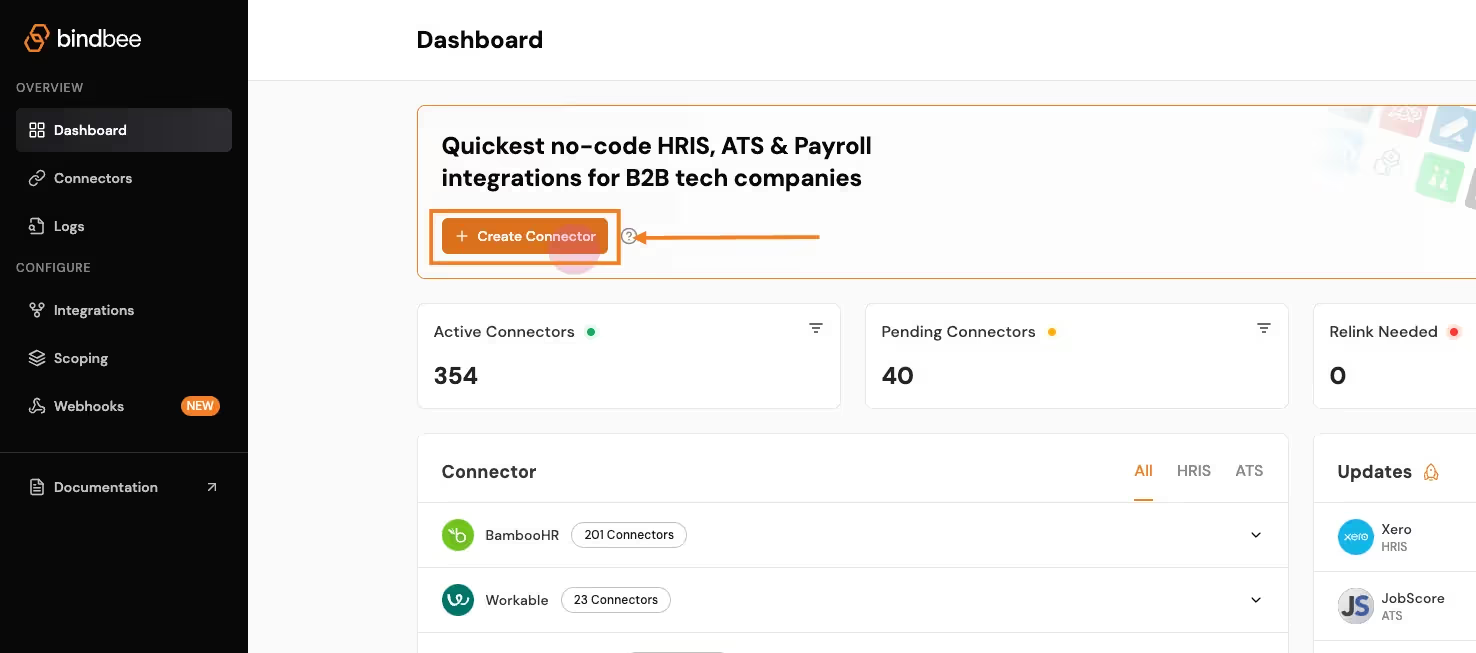
- Create a Connector:
- Click on Create Connector from the dashboard.
- Select HRIS as the type of integration. Enter customer details and give your connector a unique ID (e.g., Bob_Integration).
- Generate a Magic Link:
- After setting up the connector, click Create Link to generate a magic link. This will allow the customer to authenticate the connection with Bob API.
- Open the link and enter the necessary credentials (e.g., Bob key, subdomain). This step establishes the connection between the platform and Bindbee.
- Sync the Connector:
- Once the connection is made, the connector will begin syncing data from Bob. This may take a few minutes depending on the size of the data. You can track the sync status in the connector section.
- Access the Synced Data:
- After syncing, go to the Employee section in the Bindbee dashboard and select Get Employees to retrieve employee data from Bob.
- Get the API Key and Connector Token:
- Copy the API key and the x-connector-token from the Bindbee dashboard, which you will need to make authenticated API requests.
Retrieving Employee Data with Bindbee
Once the Deel data has been synced, you can retrieve employee data on to your application via the Bindbee API.
Here’s a step-by-step process for accessing synced data from Deel through Bindbee’s unified API:
- Request Setup:
- Use the Bindbee API to send a request for employee data. You’ll need both your Bindbee API token and the x-connector-token.
- Example Request:
- Using Python:
import requests
url = "https://api.bindbee.com/hris/v1/employees"
headers = {
"Authorization": "Bearer YOUR_BINDBEE_API_KEY",
"x-connector-token": "YOUR_CONNECTOR_TOKEN",
"Accept": "application/json"
}
response = requests.get(url, headers=headers)
print(response.json())
- Using cURL:
curl --request GET \
--url https://api.bindbee.com/hris/v1/employees \
--header 'Authorization: Bearer YOUR_BINDBEE_API_KEY' \
--header 'x-connector-token: YOUR_CONNECTOR_TOKEN'
This request will return a list of employee objects, including details like the employee’s first name, last name, job title, department, and contact information.
Sample Response:
{
"items": [
{
"id": "018b18ef-c487-703c-afd9-0ca478ccd9d6",
"first_name": "John",
"last_name": "Doe",
"job_title": "Chief Technology Officer",
"department": "Engineering",
"work_email": "john@johndoe.dev"
}
]
}
Bulk Employee Data Retrieval
For retrieving large datasets, Bindbee simplifies the process by allowing you to fetch bulk employee data from Bob.
The pagination feature helps manage large responses by returning results in pages.
What is Pagination?
“Pagination is the process of dividing a large dataset into smaller, manageable chunks or pages, allowing users to navigate through the data more easily without overwhelming them with too much information at once.”
- Pagination Parameters:
- Use the
cursor
andpage_size
parameters to navigate through the results. By default, Bindbee returns 50 records per page. - Example of a paginated request:
- Use the
url = "https://api.bindbee.com/hris/v1/employees?cursor=MDE4YjE4ZWYtYzk5Yy03YTg2LTk5NDYtN2I3YzlkNTQzM2U1&page_size=50"
response = requests.get(url, headers=headers)
- Querying for Specific Employee Data:
- You can further refine your request by filtering based on specific fields, such as manager_id, remote_id, or company_id to get employees under a particular manager or company.
Check out the entire documentation here - Bindbee’s API documentation.
Get Started with Bob API Using Bindbee
Integrating with Bob shouldn’t be an engineering battle.
Yet, for most teams, it feels like a huge time sink—draining valuable engineering resources.

Let us handle the heavy lifting. You focus on growth, what say?
Book a demo with our experts today.